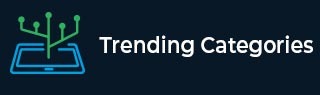
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create a border pane using JavaFX?
Once you create all the required nodes for your application you can arrange them using a layout. Where a layout is a process of calculating the position of objects in the given space. JavaFX provides various layouts in the javafx.scene.layout package.
Border Pane
In this layout, the nodes are arranged in the top, center, bottom, left, and, right positions. You can create a border pane in your application by instantiating the javafx.scene.layout.BorderPane class.
There are five properties of this class (Node type) specifying the positions in the pane, namely, top, bottom, right, left, center. You can set nodes as values to these properties using the setTop(), setBottom(), setRight(), setleft() and, setCenter().
You can set the size of the border pane using the setPrefSize() method. To add nodes to this pane you can either pass them as arguments of the constructor or, add them to the observable list of the pane as −
getChildren().addAll();
Example
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.BorderPane; import javafx.stage.Stage; public class BorderPaneExample extends Application { public void start(Stage stage) { //Creating buttons Button left = new Button("Left"); left.setPrefSize(200, 100); Button right = new Button("Right"); right.setPrefSize(200, 100); Button top = new Button("Top"); top.setPrefSize(595, 100); Button bottom = new Button("Buttom"); bottom.setPrefSize(595, 100); Button center = new Button("Center"); center.setPrefSize(200, 100); //Creating the border pane BorderPane pane = new BorderPane(); //Setting the top, bottom, center, right and left nodes to the pane pane.setTop(top); pane.setBottom(bottom); pane.setLeft(left); pane.setRight(right); pane.setCenter(center); //Setting the Scene Scene scene = new Scene(pane, 595, 300); stage.setTitle("Border Pane"); stage.setScene(scene); stage.show(); } public static void main(String args[]){ launch(args); } }