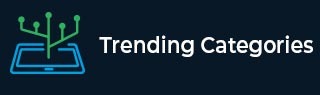
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to create a Border, Border radius, and shadow to a UIView in iPhone/iOS?
In this article we’ll learn how to create borders and shadows. We’ll do it in two ways, one by directly coding and one by making it designable and an extension of UIView, which can be edited directly in the storyboard.
Let’s see how to play around with borders in ios −
Method 1 − Creating borders with simple coding –
Borders are a property of layer, above which a View is drawn, a border has the following properties, Border color, border width.
self.view.layer.borderColor = colorLiteral(red: 0.4392156899, green: 0.01176470611, blue: 0.1921568662, alpha: 1) self.view.layer.borderWidth = 5.0
To create corner radius of a view we can use
self.view.layer.cornerRadius = 5
Here is the result of above code when run on simulator.
To create shadow we can use other properties like shadowPath, shadowColor, shadowOffSet, shadowOpactiy and shadowRadius.
Method 2 − Using Designable we can make these properties editable from the storyboard. Let’s see an example of playing around with borders using designables.
extension UIView { @IBInspectable var cornerRadius: CGFloat { get { return layer.cornerRadius } set { layer.cornerRadius = newValue } } @IBInspectable var borderWidth: CGFloat { get { return layer.borderWidth } set { layer.borderWidth = newValue } } @IBInspectable var borderColor: UIColor? { get { if let color = layer.borderColor { return UIColor(cgColor: color) } return nil } set { if let color = newValue { layer.borderColor = color.cgColor } else { layer.borderColor = nil } } } }
This will create actions in the storyboard’s Attribute Inspector from where you can directly edit and access the results. It should look like the one below.