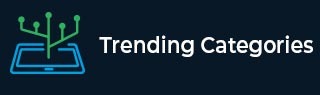
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to Create a Bootstrap Spinner and Display on Screen till the data from the API loads ?
Using Bootstrap, we will create a Bootstrap spinner and display it on the screen until data from an API is loaded. This will give customers visual indications that something is going on in the background and can be an excellent method to improve the user experience. In this blog, we will be utilizing Bootstrap, a famous front-end programming framework.
Follow the below steps to create a Bootstrap spinner and display on screen till the data from the API loads -
Step 1: Include the Bootstrap CSS and JavaScript Files
We will begin with our first step by importing JavaScript (jQuery.min.js) and CSS file(bootstrap.min.css) files in the index.html file. The link and script tag to be used are written below. Please copy and paste these lines of code in your head section of the HTML file.
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js "></script>
Step 2: Create the Spinner Element
Now comes our next step i.e., adding a div element in the body part of the index.html file. We will provide a class as “text-center” and id as spinner. We will aiso use a “ i “tag which will have the bootstrap classes for the spinner i.e., fa, fa-spinner, fa-spin and fa-3x. We are using four classes here just to be intact with the responsiveness of the page.
<div class="text-center" id="spinner"> <i class="fa fa-spinner fa-spin fa-3x"></i> </div>
Step 3: Hide the Spinner by Default
Our third step in creating a spinner is, making a spinner hidden by default. We don’t want the spinner to be visible all the time. The spinner must be visible only when the data loads with API.
To do so we will use the id selector and give it a property of display:none.
#spinner { display: none; }
Step 4: Show the Spinner when data is being loaded
Our fourth step is to show the spinner until data gets loaded. We will simply use a function called show() to do so.
$("#spinner").show();
Step 5: Hide the Spinner when data has been loaded
Our next step is to hide the spinner once data gets completely loaded. We will simply use a function called hide() to do so.
$("#spinner").hide();
Step 6: Call the API and Show Spinner
In this step, we will integrate all three steps above to generate a spinner which will show the spinner while loading the data and it will automatically hide once the data is loaded.
$.ajax({ url: "api_url", method: "GET", beforeSend: function() { $("#spinner").show(); }, success: function(data) { // do something with the data }, complete: function() { $("#spinner").hide(); } });
While submitting a GET call to the supplied API url, the spinner is displayed before the request is submitted and is then removed after it has been delivered. The data supplied by the API should be handled in the success method, for example, by showing it on the screen.
Note − To contact the APIs and display the spinner, you may also use the javascript Fetch API method rather than the $.ajax method.
Personalise the Spinner
After successfully creating the spinner and making it fully functional. We can customise it by usng the font Awesome library that can be used to generate the spinning symbol in this tutorial. YWe can also modify the spinner's icon, size, colour, and other CSS attributes.
To adjust the size of the spinner, just replace the "fa-3x" class to "fa-4x" or "fa-5x". We can also alter the colour of the spinner by adding a new class to the I element and declaring it in the CSS file.
Now, let’s combine all of our work in the index.html file below.
<!DOCTYPE html> <html> <head> <title>Bootstrap Spinner Tutorial</title> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous" /> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" /> <style> #spinner { position: absolute; top: 50%; left: 50%; width: 3.5rem; height: 3.5rem; /* transform: translate(-50%, -50%); */ display: none; } </style> </head> <body> <div class="spinner-border m-5" role="status" id="spinner"> <span class="visually-hidden">Loading...</span> </div> <div id="dataContainer"></div> <script> function getData() { var spinner = document.getElementById("spinner"); spinner.style.display = "block"; setTimeout(function () { fetch("https://jsonplaceholder.typicode.com/posts") .then((response) => response.json()) .then((data) => { data.forEach((item) => { var div = document.createElement("div"); var p = document.createElement("p"); p.innerText = item.title; div.appendChild(p); document.getElementById("dataContainer").appendChild(div); }); spinner.style.display = "none"; }); }, 5000); } getData(); </script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM" crossorigin="anonymous" ></script> </body> </html>
In this tutorial, we started with the introduction of adding required files i.e., js and css in our index file. Then we created the spinner using bootstrap library and made it function using jquery library. We also discussed customising the spinner using the font awesome library. We finally concluded our discussion by including all our codes in the index.html file.