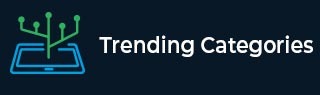
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to convert a value to a safe integer using JavaScript?
An integer is known to be a safe integer if that can be exactly represented as an IEEE-754 double precision number. A safe integer is an integer in the range -(2^53 - 1) to (2^53 - 1). All these numbers are considered safe integer because it allows the one-to-one mapping of the numbers between the mathematical integers and their representation in JavaScript.
Syntax
Following the syntax to check for a safe integer −
Number.isSafeInteger(testValue)
Here testValue is the number to check if it is a safe integer or not.
It will return true if testValue is a safe integer else false.
Example
Check If a Number is a Safe Integer
We can check whether a number is a safe integer or not by using the function isSafeInteger() as shown in the example below.
<html> <body> <p id="first"> </p> <p id="second"> </p> <p id="third"> </p> <script> //var number= 3; let result1=Number.isSafeInteger(3); let result2=Number.isSafeInteger(3.2); let result3=Number.isSafeInteger(Math.pow(2, 53)); document.getElementById("first").innerHTML = result1; document.getElementById("second").innerHTML = result2; document.getElementById("third").innerHTML = result3; </script> </body> </html>
Here we can see in the output we are getting values as true for number 3, as false for number 3.2, and for number 2^53.
Note − The Number.isSafeInteger() function is an ECMAScript6 (ES6) feature so it is supported in all modern browsers except Internet Explorer 11 or earlier.
Algorithm (Convert a Number to a Safe Integer)
To convert a number into a safe integer, we are taking one input of any unsafe integer and converting it into a safe number.
Step 1 − Store the inputted unsafe integer to a variable named “number”.
Step 2 − Find the maximum safe integer of the number. To find the maximum safe integer we use the function MAX_SAFE_INTEGER and store it in a variable named “max”.
Step 3 − Find the minimum safe integer of the above calculated maximum safe integer max. To find the minimum safe integer we use the function MIN_SAFE_INTEGER and store it in a variable named “min”.
Step 4 − Take a round off of the value min and store it in a variable named “safeInt”.
Following is the Code snippet of the above steps −
const number = prompt('Please enter any unsafe integer:'); var max = Math.min(number, Number.MAX_SAFE_INTEGER); var min = Math.max(max, Number.MIN_SAFE_INTEGER); const safeInt = Math.round(min);
Example 2
We can convert a number into a safe integer by using the example shown below example below −
<!DOCTYPE html> <html> <body> <h3> Convert a Number to a Safe Integer </h3> <p class="1"> Click the button below to take an integer as input and convert it to a safe integer.</p> <button onclick="check()">click me</button> <p id="2"></p> <script> function check(){ const number = prompt('Please enter any unsafe integer:'); var max = Math.min(number,Number.MAX_SAFE_INTEGER); var min = Math.max(max,Number.MIN_SAFE_INTEGER); const safeInt = Math.round(min); document.getElementById("2").innerHTML = number + " => " + safeInt; } </script> </body> </html>
As shown in the output window after clicking the “click me” button, the window will ask you to enter any unsafe integer.
After entering any unsafe integer in the prompt box you will get it converted into a safe integer let’s take an unsafe number 6.2 as an example.