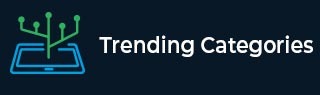
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to convert a string to int in Lua programming?
Lua does the implicit conversion or also known as coercion when it notices that you are trying to use a number but wrote a string, then it automatically converts the string into an int, and it is quite useful.
Let’s consider a simple example where I will declare a string variable, and then I will try to do an arithmetic operation on it, then once the Lua compiler infers that we are trying to use the string as an int, it will automatically convert it into an int.
Example
Consider the example shown below −
str = "10" print(type(str)) num = 2 * str print(num) print(type(num))
Output
string 20 number
Now that we know about the automatic conversion (coercion), it’s a good idea to learn how to do the conversion explicitly, the first approach is to append a 0 to the string value, and that value will be converted to an integer.
Example
Consider the example shown below −
-- uncommon method str = "100" num = str + 0 print(type(num)) print(num)
Output
number 100
The above approach is not a very common one, and it's better to use the library function to convert a string to a number.
Example
Consider the example shown below −
-- common method str = "100" num = tonumber(str) print(type(num)) print(num)
Output
number 100