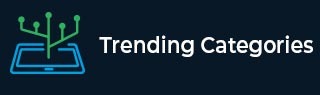
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to convert a pixel value to a number value using JavaScript?
Converting pixel values to number values in JavaScript is a simple task that can be accomplished using built-in functions such as parseInt(), parseFloat(), Number() and also string method replace(). Each method has its own advantages and disadvantages and the best method to use will depend on the specific requirements of your project.
When positioning and styling items on a web page, it's sometimes required to work with both pixel and numeric values in web development. To execute computations or operations on a value, for instance, you might need to convert a pixel value, such as "100px," to a number value, such as "100." In this article, we'll talk about using JavaScript to change a pixel value into an integer value.
Method 1: Using the parseInt() Method
A built-in JavaScript function called parseInt() can be used to translate a string into an integer. Using the parseInt() method, we can convert a string value to an integer, remove the "px" unit from the string, and then convert the integer back to a numeric value.
Example
<html> <body> <p id="result1"></p> <p id="result2"></p> <script> let pixelValue = "100px"; document.getElementById("result1").innerHTML = "Pixel value: " + pixelValue ; let numberValue = parseInt(pixelValue); document.getElementById("result2").innerHTML = "Number value: " + numberValue; </script> </body> </html>
Method 2: Using the replace() Method
A stated value can be replaced with another value using the replace() method, which is a string method. By removing the "px" unit from the string using the replace() method, we may change a pixel value to a number value.
Example
<html> <body> <p id="result1"></p> <p id="result2"></p> <script> let pixelValue = "100px"; document.getElementById("result1").innerHTML = "Pixel value: " + pixelValue ; let numberValue = parseInt(pixelValue.replace("px", "")); document.getElementById("result2").innerHTML = "Number value: " + numberValue; </script> </body> </html>
Method 3: Using the parseFloat() Method
Similar to the parseInt() method, the parseFloat() method turns a string into a float. The parseFloat() method can be used to transform a textual value from a float to an integer value, and the "px" unit can subsequently be removed.
Example
<html> <body> <p id="result1"></p> <p id="result2"></p> <script> let pixelValue = "100.5px"; document.getElementById("result1").innerHTML = "Pixel value: " + pixelValue ; let numberValue = parseFloat(pixelValue.replace("px", "")); document.getElementById("result2").innerHTML = "Number value: " + numberValue; </script> </body> </html>
Method 4: Using the Number() constructor
The Number() constructor function creates a Number object that wraps the value passed in.
Example
<html> <body> <p id="result1"></p> <p id="result2"></p> <script> let pixelValue = "100px"; document.getElementById("result1").innerHTML = "Pixel value: " + pixelValue ; let numberValue = Number(pixelValue.replace("px", "")); document.getElementById("result2").innerHTML = "Number value: " + numberValue; </script> </body> </html>
In order to match only the px unit and not any substring that contains the 'px' sequence, it is safer to use a regular expression as the first parameter when using the replace() method. like this −
Example
Another important note is that, if the pixel value passed is not a string with a "px" in the end, those methods will return NaN (Not a Number). It's important to add validation to your code to handle such cases.
<html> <body> <p id="result1"></p> <p id="result2"></p> <script> let pixelValue = "100px"; document.getElementById("result1").innerHTML = "Pixel value: " + pixelValue ; let numberValue = parseInt(pixelValue.replace(/px/g, "")); document.getElementById("result2").innerHTML = "Number value: " + numberValue; </script> </body> </html>