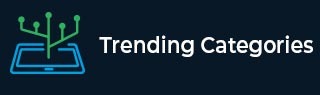
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to convert a Date object in to Timestamp in JDBC program?
The getTime() method of the java.sql.Date class retrieves and returns the time from the current timestamp in milliseconds (long) from epoch time 1, 1970 00:00:00.000 GMT.
//Retrieving the date Date date = rs.getDate("Dispatch_Date");
The constructor of the java.sql.Timestamp class accepts a long variable representing the time in milliseconds from the epoch time and constructs the Timestamp object.
//Creating a Timestamp object. Timestamp ts = new Timestamp(date.getTime()));
Using these, you can convert a Date object to TimeStamp object in JDBC.
Assume we have established connection with MySQL database and created a table named dispatch_data using statement object as:
Assume we have established connection with MySQL database and created a table named dispatch_data using statement object as:
//Creating a Statement object Statement stmt = con.createStatement(); //Query to create a table String create_query = "Create table dispatch_data (" + "Product_Name VARCHAR(255), " + "Name_Of_Customer VARCHAR(255) , " + "Dispatch_Date date, " + "Location VARCHAR(255) )"; stmt.execute(create_query); System.out.println("table created......");
We have populated the table using PreparedStatement as:
//Inserting values to a table String query = "INSERT INTO dispatch_data VALUES (?, ?, ?, ?)"; PreparedStatement pstmt = con.prepareStatement(query); pstmt.setString(1, "KeyBoard"); pstmt.setString(2, "Amith"); pstmt.setDate(3, new Date(376401869000L)); pstmt.setString(4, "Hyderabad"); pstmt.execute(); pstmt.setString(1, "Ear phones"); pstmt.setString(2, "Sumith"); pstmt.setDate(3, new Date(356788333000L)); pstmt.setString(4, "Vishakhapatnam"); pstmt.execute(); pstmt.setString(1, "Mouse"); pstmt.setString(2, "Sudha"); pstmt.setDate(3, new Date(594733933000L)); pstmt.setString(4, "Vijayawada"); pstmt.execute(); System.out.println("Records inserted......");
Following JDBC program retrieves the date values from the ResultSet converts into Timestamp object and prints the details.
import java.sql.Connection; import java.sql.Date; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.Statement; import java.sql.Timestamp; public class DateToTimeStamp { public static void main(String args[])throws Exception { //Registering the Driver DriverManager.registerDriver(new com.mysql.jdbc.Driver()); //Getting the connection String mysqlUrl = "jdbc:mysql://localhost/mydatabase"; Connection con = DriverManager.getConnection(mysqlUrl, "root", "password"); System.out.println("Connection established......"); //Creating a Statement object Statement stmt = con.createStatement(); //Creating Statement object stmt = con.createStatement(); ResultSet rs = stmt.executeQuery("select * from dispatch_data"); //Retrieving values while(rs.next()) { System.out.println("Product Name: "+rs.getString("Product_Name")); System.out.println("Name Of The Customer: "+rs.getString("Name_Of_Customer")); //Retrieving the date Date date = rs.getDate("Dispatch_Date"); //Printing the time of dispatch System.out.println("Dispatch_Timestamp: "+new Timestamp(date.getTime())); System.out.println(); } } }
Output
Connection established...... Product Name: KeyBoard Name Of The Customer: Amith Dispatch_Timestamp: 1981-12-05 00:00:00.0 Product Name: Ear phones Name Of The Customer: Sumith Dispatch_Timestamp: 1981-04-22 00:00:00.0 Product Name: Mouse Name Of The Customer: Sudha Dispatch_Timestamp: 1988-11-05 00:00:00.0