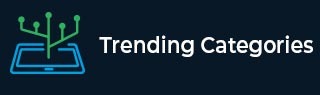
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to concatenate two strings in Golang?
The simplest way to concatenate two strings in Golang is to use the "+" operator. For example,
Example 1
package main import ( "fmt" ) func main() { str1 := "Hello..." str2 := "How are you doing?" fmt.Println("1st String:", str1) fmt.Println("2nd String:", str2) // Concatenate using the + Operator fmt.Println("Concatenated String:", str1 + str2) }
Output
It will produce the following output
1st String: Hello... 2nd String: How are you doing? Concatenated String: Hello...How are you doing?
Concatenate using strings.Join()
strings.Join() is a built-in function in Golang which is used to concatenate a slice of strings into a single string.
Syntax
Its syntax is as follows −
func Join(stringSlice []string, sep string) string
Where,
- stringSlice – The strings to be concatenated.
- sep – It is a separator string that is to be placed between the slice elements.
Example 2
Let us consider the following example −
package main import ( "fmt" "strings" ) func main() { // Initializing the Strings m := []string{"IndexByte", "String", "Function"} n := []string{"Golang", "IndexByte", "String", "Package"} // Display the Strings fmt.Println("Set 1 - Slices of Strings:", m) fmt.Println("Set 2 - Slices of Strings:", n) // Using the Join Function output1 := strings.Join(m, "-") output2 := strings.Join(m, "/") output3 := strings.Join(n, "*") output4 := strings.Join(n, "$") // Display the Join Output fmt.Println("\n Joining the slices of Set 1 with '-' delimiter: \n", output1) fmt.Println("\n Joining the slices of Set 1 with '/' delimiter: \n", output2) fmt.Println("\n Joining the slices of Set 2 with '*' delimiter: \n", output3) fmt.Println("\n Joining the slices of Set 2 with '$' delimiter: \n", output4) }
Output
It will generate the following output −
Set 1 - Slices of Strings: [IndexByte String Function] Set 2 - Slices of Strings: [Golang IndexByte String Package] Joining the slices of Set 1 with '-' delimiter: IndexByte-String-Function Joining the slices of Set 1 with '/' delimiter: IndexByte/String/Function Joining the slices of Set 2 with '*' delimiter: Golang*IndexByte*String*Package Joining the slices of Set 2 with '$' delimiter: Golang$IndexByte$String$Package
Example 3
Let us take another example.
package main import ( "fmt" "strings" ) func main() { // Defining the Variables var s []string var substr string var substr1 string var result string var output string // Intializing the Strings s = []string{"This", "is", "String", "Function"} substr = "..." substr1 = " " // Display the input slice of strings fmt.Println("Input Slice of Strings:", s) // Using the Join Function result = strings.Join(s, substr) output = strings.Join(s, substr1) // Displaying output of Join function fmt.Println("Joining with '...' delimiter:", result) fmt.Println("Joining with ' ' delimiter:", output) }
Output
It will generate the following output −
Input Slice of Strings: [This is String Function] Joining with '...' delimiter: This...is...String...Function Joining with ' ' delimiter: This is String Function
Advertisements