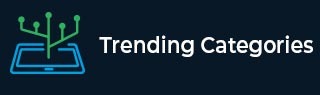
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to combine 2 arrays into 1 object in JavaScript
Let’s say, we have two arrays of equal lengths and are required to write a function that maps the two arrays into an object. The corresponding elements of the first array becomes the corresponding keys of the object and the elements of the second array become the value.
We will reduce the first array, at the same time accessing elements of the second array by index. The code for this will be −
Example
const keys = [ 'firstName', 'lastName', 'isEmployed', 'occupation', 'address', 'salary', 'expenditure' ]; const values = [ 'Hitesh', 'Kumar', false, 'Frontend Developer', 'Tilak Nagar, New Delhi', 90000, 45000 ]; const combineArrays = (first, second) => { return first.reduce((acc, val, ind) => { acc[val] = second[ind]; return acc; }, {}); }; console.log(combineArrays(keys, values));
Output
The output in the console will be −
{ firstName: 'Hitesh', lastName: 'Kumar', isEmployed: false, occupation: 'Frontend Developer', address: 'Tilak Nagar, New Delhi', salary: 90000, expenditure: 45000 }
Advertisements