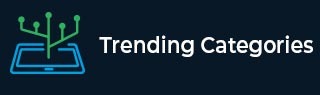
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to click on a hyper link using linkText in Selenium?
A hyperlink on a page is identified with the anchor tag. To click a link, we can use the link text locator which matches the text enclosed within the anchor tag.
We can also use the partial link text locator which matches the text enclosed within the anchor tag partially. NoSuchElementException is thrown if there is no matching element found by both these locators.
Syntax
WebElement t =driver.findElement(By.partialLinkText("Refund")); WebElement m =driver.findElement(By.linkText("Refund Policy"));
Let us make an attempt to click a link Refund Policy on the below page −
Example
Code Implementation with linkText
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import java.util.concurrent.TimeUnit; public class LnkClick{ public static void main(String[] args) { System.setProperty("webdriver.gecko.driver", "C:\Users\ghs6kor\Desktop\Java\geckodriver.exe"); WebDriver driver = new FirefoxDriver(); //implicit wait driver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS); //URL launch driver.get("https://www.tutorialspoint.com/about/about_careers.htm"); // identify element with link text WebElement m =driver.findElement(By.linkText("Refund Policy")); m.click(); System.out.println("Page navigated: " + driver.getTitle()); driver.quit(); } }
Code Implementation with partialLinkText
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.firefox.FirefoxDriver; import java.util.concurrent.TimeUnit; public class PartialLnkClick{ public static void main(String[] args) { System.setProperty("webdriver.gecko.driver", "C:\Users\ghs6kor\Desktop\Java\geckodriver.exe"); WebDriver driver = new FirefoxDriver(); //implicit wait driver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS); //URL launch driver.get("https://www.tutorialspoint.com/about/about_careers.htm"); // identify element with partial link text WebElement p =driver.findElement(By.partialLinkText("Refund")); p.click(); System.out.println("Page navigated: " + driver.getTitle()); driver.quit(); } }
Output
Advertisements