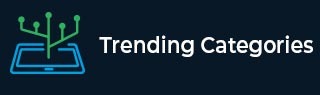
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to check whether the Pandas series is having Nan values or not?
To check whether the pandas series object is having null values or not, we can use the “hasans” attribute.
The “hasnans” is a pandas attribute that is used to identify if there any null values are present in the given series object. Generally, it returns a boolean output as a result. It returns True if there are anyone or more NaN values, or otherwise, it will return False.
This panda “hasnans” property is very similar to the pandas methods like Isnull(), isna(). These methods are used to return an array with boolean values which are used to represent the null values.
By using this “hasnans” attribute we will get a single boolean value as an output to check if the series object contains any NaN values or not.
Example 1
import pandas as pd import numpy as np # create a sample series s = pd.Series([1.2,43, np.nan,5.65,np.nan]) print(s) # applying hasnans attribute print("Output:", s.hasnans)
Explanation
Let’s take an example and apply the “hasnans” attribute to identify the nan values of a series object. Firstly, we have to import the NumPy module to initialize a series with null values. And then apply the hasnans property on the series object to observe the results.
Output
0 1.20 1 43.00 2 NaN 3 5.65 4 NaN dtype: float64 Output: True
In the above block, we can see the output of the hasnans attribute which is “True”. That means the “hasnans” attribute identifies the NaN values present in a given series object.
Example 2
import pandas as pd import numpy as np # create a sample series s = pd.Series([1.2,2,3,4,5]) print(s) # applying hasnans attribute print("Output:", s.hasnans)
Explanation
Let’s take another example without NaN values and observe the output, initially create a simple pandas series object.
Output
0 1.2 1 2.0 2 3.0 3 4.0 4 5.0 dtype: float64 Output: False
The pandas series object is created by using a python list with integer values of length 5, And there are no NaN values in it. We can observe a Boolean value “False” in the above output block which is resultant from the “hasnan” attribute.