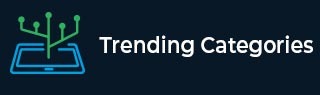
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How To Check Whether a Number is Pointer Prime Number or Not in Java?
A number is said to be a pointer prime number, if the sum of the product value of digits and the original prime number results in the next prime number.
For more clarification, we take a prime number and multiply the digits with each other and add that multiplication value with the original number. After that if we get another prime number which is next to the original prime number. Then we can say that the number is a pointer prime number.
Some examples of pointer prime numbers are: 23, 61, 1123, 1231 ... etc.
In this article, we will see how to check if a number is a pointer prime number by using Java programming language.
To show you some instances
Instance-1
Input number is 23.
Let’s check it by using the logic of the Pointer prime number.
Product of digits of 23 = 2 * 3 = 6.
Add that Value with original number= 23 + 6= 29.
The actual prime number which is next to our original number is 29.
As we notice here both the calculated number and the next prime number are the same.
Hence, 23 is a pointer prime number.
Instance-2
Input number is 1123.
Let’s check it by using the logic of the Pointer prime number.
Product of digits of 1123 =1 * 1 * 2 * 3 = 6.
Add that Value with original number= 1123 + 6= 1129.
The actual prime number which is next to our original number is 1129.
As we notice here both the calculated number and the next prime number are the same.
Hence, 1123 is a pointer prime number.
Instance-3
Input number is 147.
Let’s check it by using the logic of the Pointer prime number.
Product of digits of 147 =1 * 4 * 7 = 28.
Add that Value with original number= 147 + 28= 175.
As we notice here the calculated number is not a prime number.
Hence, 147 is not a pointer prime number.
Algorithm
Step-1 - Get the input number either by initialization or by user input.
Step-2 - Check if the input number is prime number or not.
Step-3 - Determine the prime number which is actually next to the original number.
Step-4 - Use the algorithm for calculating the pointer prime number.
Step-5 - If both the calculated value and actual next prime number are same, then the input number is called a pointer prime number else not.
Multiple Approaches
We have provided the solution in different approaches.
By Using static Input Value with user defined method
By Using user Input Value with user defined method
Let’s see the program along with its output one by one.
Approach-1: By Using Static Input Value with User Defined Method
In this approach, we declare a variable with a static input and then by using the algorithm we can check whether the number is a pointer prime number or not.
Example
public class Main { public static void main(String[] args) { // Declare a variable and store the value by static input method int inputNumber = 23; // call the function to check the pointer prime number if (checkPointerPrime(inputNumber)) System.out.print(inputNumber + " is a pointer prime number."); else System.out.print(inputNumber + " is not a pointer prime number."); } //user-defined method to calculate product value of digits static int digitProduct(int num) { int prod = 1; //initiate loop to calculate product value while (num != 0) { prod = prod * (num % 10); //remove the last digit num = num / 10; } return prod; } // user-defined method to check the prime number public static boolean checkPrim(int num) { if (num <= 1) return false; //initiate the loop for (int i = 2; i < num; i++) //if condition to check whether the number is divisible by any number or not if (num % i == 0) //if true then return false return false; //otherwise return true return true; } // user-defined function to check the number is pointer prime number or not static int nextPrimeNum(int num) { //starting phase if (num <= 1) return 2; int nextPrime = num; boolean flag = false; // loop to check continuously for prime number while (!flag) { nextPrime++; if (checkPrim(nextPrime)) flag = true; } return nextPrime; } // user-defined method to check Pointer-Prime numbers static boolean checkPointerPrime(int num) { //condition for pointer prime number if (checkPrim(num) && (num + digitProduct(num) == nextPrimeNum(num))) return true; else return false; } }
Output
23 is a pointer prime number
Approach-2: By Using User Input Value with User Defined Method
In this approach, we ask the user to enter a number as input number and pass this number as a parameter in a user defined method, then inside the method by using the algorithm we can check whether the number is a pointer prime number or not.
Example
import java.util.Scanner; public class Main { public static void main(String[] args) { //create object of Scanner class Scanner sc=new Scanner(System.in); //ask user to give the input System.out.print("Enter a number: "); //declare a variable and store the input value int inputNumber=sc.nextInt(); // call the function to check the pointer prime number if (checkPointerPrime(inputNumber)) System.out.print(inputNumber + " is a pointer prime number."); else System.out.print(inputNumber + " is not a pointer prime number."); } //user-defined method to calculate product value of digits static int digitProduct(int num) { int prod = 1; //initiate loop to calculate product value while (num != 0) { prod = prod * (num % 10); //remove the last digit num = num / 10; } return prod; } // user-defined method to check the prime number public static boolean checkPrim(int num) { if (num <= 1) return false; //initiate the loop for (int i = 2; i < num; i++) //if condition to check whether the number is divisible by any number or not if (num % i == 0) //if true then return false return false; //otherwise return true return true; } // user-defined function to check the number is pointer prime number or not static int nextPrimeNum(int num) { //starting phase if (num <= 1) return 2; int nextPrime = num; boolean flag = false; // loop to check continuously for prime number while (!flag) { nextPrime++; if (checkPrim(nextPrime)) flag = true; } return nextPrime; } // user-defined method to check Pointer-Prime numbers static boolean checkPointerPrime(int num) { //condition for pointer prime number if (checkPrim(num) && (num + digitProduct(num) == nextPrimeNum(num))) return true; else return false; } }
Output
Enter a number: 1123 1123 is a pointer prime number
In this article, we explored how to check a number whether it is a pointer prime number or not in Java by using different approaches.