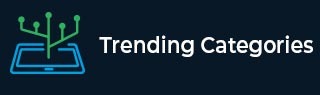
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to check if an element is visible with WebDriver?
We can check if an element exists with Selenium webdriver. There are multiple ways to check it. We shall use the explicit wait concept in synchronization to verify the visibility of an element.
Let us consider the below webelement and check if it is visible on the page. There is a condition called visibilityOfElementLocated which we will use to check for element visibility. It shall wait for a specified amount of time for the element after which it shall throw an exception.
We need to import org.openqa.selenium.support.ui.ExpectedConditions and import org.openqa.selenium.support.ui.WebDriverWait to incorporate expected conditions and WebDriverWait class. We will introduce a try/catch block. In the catch block, we shall throw the NoSuchElementException in case the element is not visible on the page.
We can also confirm if an element is visible with the help of isDisplayed() method.This method returns a true or a false value. In case the element is invisible, the method returns a false value.
Let us check if the below element is visible−
Example
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; import org.openqa.selenium.support.ui.WebDriverWait; import org.openqa.selenium.support.ui.ExpectedConditions; import java.util.NoSuchElementException; public class CheckVisibile{ public static void main(String[] args) { System.setProperty("webdriver.chrome.driver","C:\Users\ghs6kor\Desktop\Java\chromedriver.exe"); WebDriver driver = new ChromeDriver(); driver.manage().timeouts().implicitlyWait(8, TimeUnit.SECONDS); driver.get("https://www.tutorialspoint.com/index.htm"); try { // identify element WebElement t = driver.findElement(By.cssSelector("h4")); // Explicit wait condition for visibility of element WebDriverWait w = new WebDriverWait(driver, 5); w.until(ExpectedConditions .visibilityOfElementLocated(By.cssSelector("h4"))); System.out.println("Element is visible"); } catch(NoSuchElementException n) { System.out.println("Element is invisible"); } driver.close(); } }
Example
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class CheckIsDisplayed{ public static void main(String[] args) { System.setProperty("webdriver.chrome.driver","C:\Users\ghs6kor\Desktop\Java\chromedriver.exe"); WebDriver driver = new ChromeDriver(); driver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS); driver.get("https://www.tutorialspoint.com/index.htm"); // identify element try { WebElement t = driver.findElement(By.cssSelector("h4")); // check visibility with isDisplayed() if (t.isDisplayed()){ System.out.println("Element is visible"); } catch(Exception n) { System.out.println("Element is invisible"); } driver.close(); } }