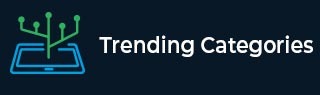
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to check if a "lateInit" variable has been initialized in Kotlin?
Any variable which is initialized after its declaration is known as a "late initialized variable". In conventional programming languages, any non-NULL type of variable need to be initialized in the constructor. But sometimes, by mistake, developers forget to do these NULL checks which causes a programming error. In order to avoid this situation, Kotlin introduced a new modifier called as "lateInit". Along with this modifier, Kotlin provides a couple of methods to check whether this variable is initialized or not.
In order to create a "lateInit" variable, we just need to add the keyword "lateInit" as an access modifier of that variable. Following are the couple of conditions that need to be followed in order to use "lateInit" in Kotlin −
Use "lateInit" with a mutable variable. That means, we need use "var" keyword with "lateInit".
"lateInit" is only allowed with non-NULLable data types.
"lateInit" does not work with primitive data types.
"lateInit" can be used when the variable property does not have any getter and setter methods.
Example
In this example, we will declare a variable as a "lateInit" variable and we will be using our Kotlin library function to check whether the variable is initialized or not.
class Tutorial { lateinit var name : String fun checkLateInit(){ println(this::name.isInitialized) // it will print false as the value is not initialized // initializing name name = "www.tutorialspoint.com/" println(this::name.isInitialized) // It will return true } } fun main() { var obj=Tutorial(); obj.checkLateInit(); }
Output
Once you execute the code, it will generate the following output −
false true
In the second case, the variable name is initialized, hence it returns True.