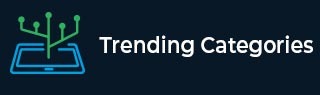
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to check if a file is readable, writable, or, executable in Java?
In general, whenever you create a file you can restrict/permit certain users from reading/writing/executing a file.
In Java files (their abstract paths) are represented by the File class of the java.io package. This class provides various methods to perform various operations on files such as read, write, delete, rename, etc.
In addition, this class also provides the following methods −
setExecutble() − This method issued to set the execute permissions to the file represented by the current (File) object.
setWritable() − This method is used to set the write permissions to the file represented by the current (File) object.
setReadable() − This method is used to set the read permissions to the file represented by the current (File) object.
Example
Following Java program creates a file writes some data into It and set read, write and execute permission to it.
import java.io.File; import java.io.FileWriter; import java.io.IOException; public class FilePermissions { public static void main(String args[]) throws IOException { String filePath = "D:\sample.txt"; //Creating a file File file = new File(filePath); System.out.println("File created........."); //Writing data into the file FileWriter writer = new FileWriter(file); String data = "Hello welcome to Tutorialspoint"; writer.write(data); System.out.println("Data entered........."); //Setting permissions to the file file.setReadable(true); //read file.setWritable(true); //write file.setExecutable(true); //execute System.out.println("Permissions granted........."); } }
Output
Directory created......... File created......... Data entered......... Permissions granted.........
Readable, writable, executable
In addition to the class File of the java.io package, since Java 7 the Files class was introduced this contains (static) methods that operate on files, directories, or other types of files.
You can verify whether a particular file has read, write, execute permissions you can use the isReadable(), isWritable() and, isExecutable() methods of this class.
The isReadable() method − This method accepts an object of the Path class and verifies whether the file represented by the given path exists in the system and JVM has permission to read it. If so, it returns true else it returns false.
The isWritable() method − This method accepts an object of the Path class and verifies whether the file represented by the given path exists in the system and JVM has permission to write to it. If so, it returns true else it returns false.
The isExecutable() method − This method accepts an object of the Path class and verifies whether the file represented by the given path exists in the system and JVM has permissions to execute it. If so, it returns true else it returns false.
The Files class
The class to provides a method named exists(), which returns true if the file represented by the current object(s) exists in the system else it returns false.
Example
The following Java program verifies whether a specified file exists in the system. It uses the methods of the Files class.
import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; public class FilesExample { public static void main(String args[]) { //Creating a Path object Path path = Paths.get("D:\sample.txt"); //Verifying if the file is readable boolean bool = Files.isReadable(path); if(bool) { System.out.println("readable"); } else { System.out.println("not readable"); } bool = Files.isWritable(path); if(bool) { System.out.println("writable"); } else { System.out.println("not writable"); } bool = Files.isExecutable(path); if(bool) { System.out.println("executable"); } else { System.out.println("not executable"); } } }
Output
readable writable executable