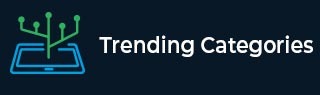
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to check a URL contains a hash or not using JavaScript?
Overview
To check whether a Uniform Resource Locator (U.R.L.) contains a hash (#text) or not with JavaScript, as JavaScript contains some pre-build methods which makes a straightforward task to obtain a certain goal. This can be done by using the hash property in JavaScript, which can be accessed by initializing the window.location object. It eases the user-interface and provides a foremost navigation through the web page.
To build this solution, we need prior knowledge on the following topics −
HTML- To build the skeleton of the page. In which we will use an internal <script/> tag.
HTML Events (onclick(), onchange(), etc.)
JavaScript window object, location object, hash property.
Syntax
The basic syntax used in this program is −
window.location.hash
Window − This is the JavaScript object that specifies the frame of the web browser. It can handle the method related to your browser. The property of windows can be accessed by the
Syntax:
(window.property/methodName)
Location − It is a property of the window object that contains information about the current web page URL.
Syntax:
window.location.propertyName
Hash − It is a property of a location object that contains the text after #. If the URL contains “/tutorialspoint/#java”. Then location.hash will return the value "java."
Algorithm
Step 1 - Create a HTML button using <button> tag
Step 2 - Insert an onclick event in the button tag <button onclick= “”> with the function in it, as <button onclick= “checkHash()”>. The Function name is user-defined so you change as per your convenience.
Step 3 - Create a JavaScript arrow function checkHash().
Step 4 - Use the window object method location and location method hash. Store the result of window.location.hash in a variable.
Step 5 - Pass the variable in the if-else as condition.
Step 6 - If the variable passed in the if-else is true then it will return “Hash found”, otherwise if the variable passed is false then it will return “Hash not found”.
Example
In the given code, it contains an HTML button that contains the “onclick()” event handler, in which the JavaScript user-defined function “checkHash()” is given. When the <button> is clicked, the checkHash() function is triggered.
<!DOCTYPE html> <html lang="en"> <head> <title>Check the Hash in URL</title> <style> body{ border: 1px solid black; text-align: center; } </style> </head> <body> <p> <strong> OUTPUT HASH- </strong> <span id="outputVal" style="padding:3px;"> </span>// Output will be shown here </p> <button onclick="checkHash()">Check URL Hash</button><br> <a href="#java">add hash(#) to url</a><br> <!-- JavaScript function starts from here --> <script> checkHash=()=>{ var h = window.location.hash; if(window.location.hash){ document.getElementById("outputVal").innerText="Hash found "+h; document.getElementById("outputVal").style.background="lightgreen"; } else { document.getElementById("outputVal").innerText="No Hash Found"; document.getElementById("outputVal").style.background="tomato"; } } </script> <!-- JavaScript function ends here --> </body> </html>
After clicking on the anchor text −
In this state, the URL (http://127.0.0.1:3000/index.html) of the web page does not contain any #text as shown in the address bar in the below image, so the window.location.hash does not store anything in the referenced variable, and thus it returns false with the output “No hash found.”
After the anchor text is clicked, the value of the HTML attribute href=“#java” is concatenated with the current URL, thus the window.location.hash contains the concatenated hash text “#java,” thus it returns true with the name of the hash stored in variable “h,” and the “h” variable will be checked in the if-else condition whose output will be shown inside the “outputVal” id container.
Conclusion
When the anchor link is clicked, it redirects us to the hash-valued content.
This provides the user with an interactive interface with directions towards the hashed linked content.The location object also has many favorable properties, such as href, origin, pathname, and many more. Window objects also provide a variety of methods that manipulate the browser, such as location, history, open(), close(), and many more.