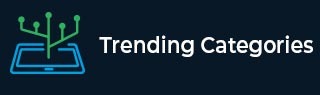
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to center a Tkinter widget in a sticky frame?
Tkinter has lots of inbuilt functions and methods that can be used configure the properties of Tkinter widgets. These properties vary with different geometry managers. The grid geometry manager is one of them which deals with many complex layout problems in any application. Grid geometry manager adds all the widgets in the given space (if applicable) without overlapping each other.
Let us suppose that we have created a sticky frame using Grid geometry manager and we want to center the Label text widget inside the frame. In this case, we have to first make the main window sticky by configuring the row and column property. Once the main window gets sticky with the frame, it can make any widget rationally resizable. The label widget must be sticky in this case. Now, to center the widget, specify the value of the row, column and weight.
Example
# Import the required library from tkinter import * # Create an instance of tkinter frame win= Tk() # Set the size of the Tkinter window win.geometry("700x350") # Add a frame to set the size of the window frame= Frame(win, relief= 'sunken') frame.grid(sticky= "we") # Make the frame sticky for every case frame.grid_rowconfigure(0, weight=1) frame.grid_columnconfigure(0, weight=1) # Make the window sticky for every case win.grid_rowconfigure(0, weight=1) win.grid_columnconfigure(0, weight=1) # Add a label widget label= Label(frame, text= "Hey Folks! Welcome to Tutorialspoint", font=('Helvetica 15 bold'), bg= "white") label.grid(row=3,column=0) label.grid_rowconfigure(1, weight=1) label.grid_columnconfigure(1, weight=1) win.mainloop()
Output
Executing the above code will display a centered Label Text lying inside the sticky frame.