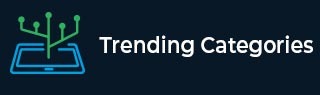
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to cancel XMLHttpRequest in AJAX?
We will use the "abort()" method to cancel the XMLHttpRequest. This will stop the current request from continuing to execute. In the future, we will make sure to properly cancel any requests that are no longer needed to optimize performance.
Let us first understand what XML HttpRequest is. XMLHttpRequest is a JavaScript object that allows web developers to make HTTP requests to a server without reloading the entire page. It is often used for creating dynamic and interactive web applications.
The API can be used to retrieve data in different formats such as text, XML, and JSON. XMLHttpRequest is supported by all modern web browsers. It is the basis of AJAX (Asynchronous JavaScript and XML) which allows for updating parts of a web page without having to reload the entire page.
Approach
To cancel an XMLHttpRequest in AJAX, you can use the abort() method of the XMLHttpRequest object. For example −
var xhr = new XMLHttpRequest(); xhr.open("GET", "example.com", true); // Cancel the request xhr.abort();
It will stop the request being sent to the server, and if the request has already been sent, it will stop the response from being processed.
You can also use the xhr.onabort event to track if the request has been canceled like this.
xhr.onabort = function() { console.log("Request cancelled"); }
It’s important to note that the abort() method only works if the request has not yet been completed. If the request has already been completed, calling abort() will have no effect.
Example
Here’s a simple example of how to cancel an XMLHttpRequest using the abort() method in JavaScript −
var xhr = new XMLHttpRequest(); xhr.open("GET", "example.php"); // Store the request in a variable var request = xhr.send(); // Later on, if you want to cancel the request request.abort();
In this example, we first create a new instance of the XMLHttpRequest object and open a GET request to an endpoint https://jsonplaceholder.typicode.com/photos.
We then store the request in a variable and send it.
If at any point we want to cancel the request, we can simply call the abort() method on the request variable.
This will immediately stop the request from being processed and prevent any further actions from taking place.
It is important to note that once an XMLHttpRequest is aborted, it cannot be restarted.
Also, it is a best practice to check the readyState property of the request before calling the abort method, as the request may already be complete and the abort method will have no effect.