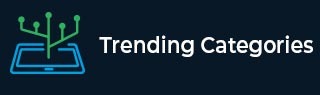
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to bind attributes in VueJS?
The v-bind directive in VueJS can be used for binding one or more attributes, or a component prop to an element. If the attribute is bonded towards our data that is defined in the Vue instance, then we can observe these data changes dynamically since the attributes are binded.
To apply the v-bind directive, we will first create a div element with id ‘app’. Once the div element is created, we can apply the v-on: click.middle directive to the element.
Syntax
We can use the following syntax to bind attributes in Vue.js −
v-bind:attribute = "expression"
Here "expression" is the value we want to bind to the attribute.
Example: Implementing v-on:click.middle directive
Create two files app.js and index.html in the Vue project. The file and directory with code snippets are given below for both files. Copy and paste the below code snipped in your Vue project and run the Vue project. You shall see the below output on the browser window.
FileName - app.js
Directory Structure -- $project/public -- app.js
// Setting the default visiblity to false var app = new Vue({ el: '#app', data: { ifActive: true } })
FileName - index.html
Directory Structure -- $project/public -- index.html
<!DOCTYPE html> <html> <head> <script src= "https://cdn.jsdelivr.net/npm/vue/dist/vue.js"> </script> <style> .active { color: blue; } .error { color: red; } </style> </head> <body> <div style="text-align: center;"> <h1 style="color: green;"> Welcome to Tutorials Point </h1> <b> v-bind directive(VueJS) </b> </div> <div id="app" style="text-align: center; padding-top: 40px;"> <button v-on:click="ifActive = !ifActive"> Click to Toggle </button> <h1 v-bind:class="{active: ifActive, error: !ifActive}"> Welcome to Tutorials Point </h1> </div> <script src='app.js'></script> </body> </html>
Run the following command to get the below output −
C://my-project/ > npm run serve
Completee Code
<!DOCTYPE html> <html> <head> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"> </script> <style> .active {color: blue;} .error {color: red;} </style> </head> <body> <div style="text-align: center;"> <h1 style="color: green;"> Welcome to Tutorials Point </h1> <b> v-bind directive(VueJS)</b> </div> <div id="app" style="text-align: center; padding-top: 40px;"> <button v-on:click="ifActive = !ifActive"> Click to Toggle </button> <h1 v-bind:class="{active: ifActive, error: !ifActive}"> Welcome to Tutorials Point </h1> </div> <script> // Setting the default visiblity to false var app = new Vue({ el: '#app', data: { ifActive: true } }) </script> </body> </html>
In this article, we demonstrated how to bind attributes in Vue.js. To perform this task we created app.js and index.html files and included app.js file in the index.html file using <script> tag. Finally, we created a complete code by combining app.js and index.html as an HTML file.