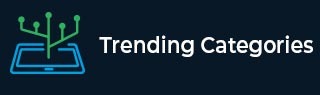
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to assign a PHP variable to JavaScript?
PHP tags with single or double quotes assign a PHP value to a JavaScript variable when setting a PHP variable. Several times you might need to put PHP variable values in JavaScript. You may easily echo on the JS variable with a PHP tag if there are only simple variables, such as a string or any integer.
Understanding the sequence of actions when you access a PHP website is necessary before passing a variable from PHP to JavaScript. To create the HTML, the PHP will first run your.php file. Your code will be delivered to the client's browser when PHP has processed it. PHP has no access to the file once it goes to the browser. JavaScript is executed after PHP because it runs within the browser.
Refer to the syntax below each method for the implementation.
Using Echo
You can use echo to transmit a PHP variable directly to JavaScript when both the PHP and JavaScript code are on the same page.
Syntax
<?php $phpvar="something"; ?> <script> const phpecho="<?php echo $phpvar; ?>"; //print </script>
JavaScript variable assigns PHP echo statement. Printing the JavaScript variable gives PHP variable value.
Example
The example works similarly to the syntax. Additionally, integer displays do not require quotes. Array display requires the json_encode function.
Index.php
<html> <body> <h2>Program to assign PHP variable value as JavaScript using <i>echo</i></h2> <?php $phpvar="I am php variable"; $intval=100; $arrval=[10, 20, 30]; ?> <b id="output"></b> <script> const phpecho="<?php echo $phpvar; ?>"; const intout=<?php echo $intval; ?>; const arrout=<?php echo json_encode($arrval); ?>; const output=document.getElementById("output"); let strvar="String = "+phpecho + "<br><br>"; strvar+= "Integer = "+intout + "<br><br>"; strvar+= "Array = "+arrout; output.innerHTML=strvar; </script> </body> </html>
Using DOM
Sometimes after a user action, you may need PHP variables. In addition to using the echo method, you can store PHP data in HTML using the DOM tag. This approach is comparable to using echo directly but is cleaner because it outputs to the DOM rather than JavaScript variables.
Syntax
<div id="color" style="display: none;"> <?php $color="Blue"; echo htmlspecialchars($color); ?> </div> <script> const color=document.getElementById("color").textContent; //print </script>
DOM assigns PHP echo statement. The script reads the DOM to get the PHP variable value.
Example
The example works similarly to the syntax. DOM assigns a string, integer, and array and displays in JavaScript.
Index.php
<html> <body> <h2>Program to assign PHP variable value as JavaScript using <i>DOM</i></h2> <div id="color" style="display: none;"> <?php $color="Blue"; echo htmlspecialchars($color); ?> </div> <div id="score" style="display: none;"> <?php $score=1000; echo htmlspecialchars($score); ?> </div> <div id="fruits" style="display: none;"> <?php $fruits=["Apple", "Orange"]; echo json_encode($fruits); ?> </div>L <b id="result"></b> <script> const color=document.getElementById("color").textContent; const score=document.getElementById("score").textContent; const fruits=document.getElementById("fruits").textContent; const result=document.getElementById("result"); result.innerHTML=color +" - "+ score+" - "+fruits; </script> </body> </html>
Using Fetch API
Use the JavaScript Fetch API if you expect to keep your JavaScript code independent from your PHP template.
Similar to AJAX and XMLHTTPRequest, Fetch API is used to send an HTTP request. You can now access resources from any location on the Internet. PHP data can be retrieved from JavaScript using Fetch.
In contrast to the other two, this approach generates an HTTP request, resulting in a delay before the data fetch.
When your PHP data needs to go through complicated processing before being sent to JavaScript, it is better to use the Fetch API.
Syntax
<script> fetch("info.php") .then((result)=> result.json()) .then((outcome)=>{//print }); </script>
Fetch API returns JSON response from another PHP file. The outcome part can print this PHP variable value.
Example
The program runs similarly to the syntax. The fetch callback stringifies the array data from info.php.
Info.php
<?php $colorarr=[ "vt"=>"Violet", "io"=>"Indigo" ]; echo json_encode($colorarr); ?>
Index.php
<html> <body> <h2>Program to assign PHP variable value as JavaScript using <i>fetch API</i></h2> <div id="display"></div> <script> fetch("info.php") .then((result)=> result.json()) .then((outcome)=>{ const display=document.getElementById("display"); display.textContent=JSON.stringify(outcome); }); </script> </body> </html>
Using a short PHP echo tag
Syntax
<?php $book='Novel'; ?> <script> var varjs='<?=$book?>'; //print </script>
JavaScript variable assigns short echo tag. Displaying this variable gives PHP variable value.
Example
The program works similarly to the echo method. The only difference is the short syntax.
Index.php
<html> <body> <h2>Program to assign PHP variable value as JavaScript using <i>short PHP echo tag</i></h2> <b id="res"></b> <?php $book='Novel'; ?> <script> var varjs='<?=$book?>'; var dom=document.getElementById("res"); dom.innerHTML=varjs; </script> </body> </html>
Using XMLHttpRequest
To transfer variables and data from the PHP server to JavaScript, we can utilize AJAX. It improves readability and makes the code cleaner.
Due to the asynchronous nature of this procedure, the webpage updates without requiring a page reload. However, latency occurs because AJAX sends the HTTP request when utilized over a network.
Syntax
<script> var request=new XMLHttpRequest(); request.onload=function() { console.log(this.responseText); }; request.open("get", "info.php", true); request.send(); </script>
XMLHttpRequest retrieves data from another PHP file. The onload function block can display the PHP variable in the response.
Example
The example executes as in the syntax. The responseText in the onload callback displays the PHP variable from info.php.
Index.php
<html> <body> <h2>Program to assign PHP variable value as JavaScript using <i>XMLHttpRequest</i></h2> <b id="out"></b> <script> var outEl=document.getElementById("out"); var request=new XMLHttpRequest(); request.onload=function() { outEl.innerHTML=this.responseText; }; request.open("get", "info.php", true); request.send(); </script> </body> </html>
Info.php
<?php $wish="Hello Index PHP. I am Info PHP"; echo json_encode($wish); ?>
Using PHP syntax inside script tag
Syntax
<?php $count='One'; ?> <script> <?php echo "var strjs ='$count';"; ?> //print </script>
JavaScript variable inside PHP syntax within the script tag contains the PHP variable value.
Example
The program runs as per the syntax. The JavaScript variable in the PHP syntax inside the script tag functions as a normal JavaScript variable. The JavaScript variable assigns the PHP variable value.
Index.php
<html> <body> <h2>Program to assign PHP variable value as JavaScript using <i>PHP syntax inside script tag</i></h2> <b id="disp"></b> <?php $count='One-Two'; ?> <script> <?php echo "var strjs ='$count';"; ?> var disEl=document.getElementById("disp"); disEl.innerHTML=strjs; </script> </body> </html>
This tutorial discussed how to assign PHP variable value as JavaScript. The echo method is simple, and you can use other methods based on the context.