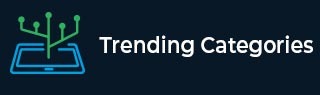
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to add styling or css to your app using reactnative?
Styling your app can be done as follows −
- Using Stylesheet component
- Using Inline styles
Using Stylesheet component
React native Stylesheet component comes very handy and neat when you want to apply styling to your app. To work with Stylesheet component first import it as shown below −
import { StyleSheet } from 'react-native';
You can create the style using Stylesheet component as follows −
const styles = StyleSheet.create({ container: { flex: 1, marginTop: StatusBar.currentHeight || 0, }, item: { margin: 10, padding: 20, marginVertical: 8, marginHorizontal: 16, } });
The above style can be used as follows inside your code −
<View style={styles.container}></View>
Here is an example that makes use of Stylesheet for display of FlatList component −
Example 1
import React from "react"; import { FlatList , Text, View, StyleSheet, StatusBar } from "react-native"; export default class App extends React.Component { constructor() { super(); this.state = { data: [ { name: "Javascript Frameworks", isTitle: true }, { name: "Angular", isTitle: false }, { name: "ReactJS", isTitle: false }, { name: "VueJS", isTitle: false }, { name: "ReactNative", isTitle: false }, { name: "PHP Frameworks", isTitle: true }, { name: "Laravel", isTitle: false }, { name: "CodeIgniter", isTitle: false }, { name: "CakePHP", isTitle: false }, { name: "Symfony", isTitle: false } ], stickyHeaderIndices: [] }; } renderItem = ({ item }) => { return ( <View style={styles.item}> <Text style={{ fontWeight: (item.isTitle) ? "bold" : "", color: (item.isTitle) ? "red" : "gray"}} > {item.name} </Text> </View> ); }; render() { return ( <View style={styles.container}> <FlatList data={this.state.data} renderItem={this.renderItem} keyExtractor={item => item.name} stickyHeaderIndices={this.state.stickyHeaderIndices} /> </View> ); } } const styles = StyleSheet.create({ container: { flex: 1, marginTop: StatusBar.currentHeight || 0, }, item: { margin: 10, padding: 20, marginVertical: 8, marginHorizontal: 16, } });
Output
Using Inline styles
You can use the style property to add the styles inline. However, this is not the best practice because it can be hard to read the code. Here is a working example on how to use inline style inside reactnative component
Example 2
export default App;
import React from 'react'; import { Button, View, Alert } from 'react-native'; const App = () => { return ( <View style={{flex :1, justifyContent: 'center', margin: 15 }}> <Button title="Click Me" color="#9C27B0" onPress={() => Alert.alert('Testing Button for React Native ')} /> </View> ); }
Output
Advertisements