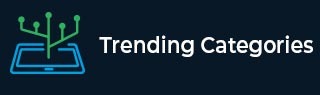
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to Add Image into Dropdown List for each items?
Creating a dropdown list with images can be a great way to make your website or application more visually appealing and user-friendly. In this article, we will go through a step-by-step example of how to add an image to each item in a dropdown list using HTML and CSS.
Approach
We will go through a step-by-step process of creating an HTML file with the necessary elements, using CSS to style and position those elements, and using the :hover selector to change the appearance of the elements when the user interacts with them. By the end of this article, you will have a solid understanding of how to add an image to each item in a dropdown list and will have a working example to reference.
Syntax
The simple syntax that we would be using to implement this would be −
.drop-content a:hover { /* styles here */ }
Example
Here is a step-by-step example of how to add an image to each item in a dropdown list using HTML and CSS.
Step 1 − Create an HTML file with a button element, which will act as the dropdown button, and a div element that will hold the dropdown content. Within the div element, create anchor elements for each item in the dropdown list and use the "img" tag to add images to each anchor element. Here is an example of the HTML code −
<!DOCTYPE html> <html> <head> <title>Adding image to drop list</title> </head> <body> <center> <h1 style="color: blue">Tutorials Point</h1> <div class="drop"> <button class="btn">Cars</button> <div class="drop-content"> <a href="#"> <img src="https://cdn-icons-png.flaticon.com/128/2330/2330453.png" width="30" height="25" /> Mercedes</a> <a href="#"> <img src="https://cdn-icons-png.flaticon.com/128/741/741407.png" width="30" height="25" /> Benz</a> <a href="#"> <img src="https://cdn-icons-png.flaticon.com/128/744/744465.png" width="30" height="25" /> Lamborghini</a> <a href="#"> <img src="https://cdn-icons-png.flaticon.com/128/1048/1048313.png" width="30" height="25" /> Porsche</a> </div> </div> </center> </body> </html>
Step 2 − Use CSS to style the button element and the div element that holds the dropdown content. In this example, we are using CSS to change the background color of the button element, give a padding to the button element, and change the font size. Here is an example of the CSS code −
btn { background-color: blue; color: red; padding: 16px; font-size: 16px; order: none; cursor: pointer; } .drop { position: relative; display: inline-block; } .drop-content { display: none; position: absolute; background-color: black; min-width: 160px; box-shadow: 0px 8px 16px 0px rgba(232, 225, 225, 0.2); z-index: 1; }
Step 3 − Use CSS to style the anchor elements that hold the images and text of the dropdown options. In this example, we are using CSS to change the color of the text, give padding to the anchor elements, and change the text decoration. Here is an example of the CSS code −
.drop-content a { color: white; padding: 12px 16px; text-decoration: none; display: block; }
Step 4 − Use the :hover selector to change the background color of the anchor element when the user hovers over it. In this example, we are using the :hover selector to change the background color to black when the user hovers over the anchor element. Here is an example of the CSS code −
.drop-content a:hover { background-color: black; }
Step 5 − Use CSS to style the dropdown button and the dropdown menu when the user hovers over them. In this example, we are using CSS to change the background color of the button to blue when the user hovers over it and to display the dropdown menu when the user hovers over the button. Here is an example of the CSS code −
.drop:hover .drop-content { display: block; } .drop:hover .btn { background-color: blue; }
Step 6 − Here is the complete code in an index.html file −
<!DOCTYPE html> <html> <head> <title>Adding image to drop list</title> <style> .btn { background-color: blue; color: red; padding: 16px; font-size: 16px; border: none; cursor: pointer; } .drop { position: relative; display: inline-block; } .drop-content { display: none; position: absolute; background-color: black; min-width: 160px; box-shadow: 0px 8px 16px 0px rgba(232, 225, 225, 0.2); z-index: 1; } drop-content a { color: white; padding: 12px 16px; text-decoration: none; display: block; } .drop-content a:hover { background-color: black; } .drop:hover .drop-content { display: block; } .drop:hover .btn { background-color: blue; } </style> </head> <body> <center> <h1 style="color: blue">Tutorials Point</h1> <div class="drop"> <button class="btn">Cars</button> <div class="drop-content"> <a href="#"> <img src="https://cdn-icons-png.flaticon.com/128/2330/2330453.png" width="30" height="25" /> Mercedes</a> <a href="#"> <img src="https://cdn-icons-png.flaticon.com/128/741/741407.png" width="30" height="25" /> Benz</a> <a href="#"> <img src="https://cdn-icons-png.flaticon.com/128/744/744465.png" width="30" height="25" /> Lamborghini</a> <a href="#"> <img src="https://cdn-icons-png.flaticon.com/128/1048/1048313.png" width="30" height="25" /> Porsch</a> </div> </div> </center> </body> </html>
Note − Here, we are using sample icons from flaticon for demonstration purposes.
Conclusion
In this article, we learned how to add an image into dropdown list for each items using different CSS property and HTML elements. Primarily, we looked at the :hover property that allows the dropdown to appear and lists out the icons in the dropdown list. We could use simple HTML and CSS elements and properties to achieve these customized designs in our webpage.