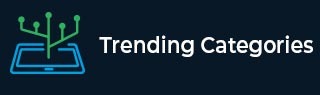
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to Add Edit and Delete Table Row in jQuery?
In this modern age of web development, effective and efficient management of tables has become important, peculiarly when dealing with data-heavy web applications. The ability to dynamically add, edit, and delete rows from a table can significantly enhance the user experience and make the application more interactive. One effective approach to achieve this is by utilizing the prowess of jQuery. jQuery provides a number of functionalities to help the developer to perform the actions.
Table Row
A table row, collection of inter-related data, is represented by the <tr> element in HTML. It is used to group cells (represented by the <td> element) together in a table. Each <tr> element is used to define a single row in the table, and usually contains one or more <td> elements for multi attribute tables.
Syntax
$(selector).append(content)
The append() method in jQuery is used to add content to the end of an element, whether it's a single element or a group of elements. The content can be text, HTML, or other elements.
Where content can be a string of HTML, a DOM element, or a jQuery object.
$(selector).find(selectCondition)
The find() function in jQuery is used to search for and select elements that are descendants of the selected element(s). It searches through the entire DOM tree of the selected element(s) and returns all matching elements in a new jQuery object.
Where selectCondition is a string representing the elements you want to select, such as a class or tag name.
$(selctor).remove()
The remove() method in jQuery is used to remove selected elements from the DOM (Document Object Model) along with their child elements.
Approach
In order to perform operations on table rows with jQuery, we'll utilize a mixture of jQuery methods and DOM manipulation techniques. Let us talk individually of all the three operations we are going to see in this article namely, add, edit and delete rows. So talking about the first operation i.e. for adding a new row to the table, we'll make use of the above mentioned append() method. To do so, first we will go about constructing a new <tr> element and then later using the append() method we will insert it into the table.
Afterwards, we'll fill the new row with new <td> elements, and assign values to them using either the text() or html() method. Now coming to the second operation i.e. modify a table row, we will first start by selecting the relevant <td> elements within the row with the find() method, and then use either the text() or html() method to modify their contents. Finally to perform the final operation i.e. t1o remove a table row, we'll just use the remove() method on the row we wish to delete.
However, before we get started with the explanation of all the parts, first I just want to bring to your notice that to include jQuery to my webpage, I have made use of the CDN which can be seen in the script tag.
Now coming back to the code, let’s talk first about the body element. The body contains a dummy table with two rows and two columns. Apart from this, it also contains three buttons labeled "Add Row," "Remove Row," and "Edit Row” which we will later use in the script to add the desired functionalities to the code. I have also added some styling using CSS to beautify the look of the table rows a little bit. Finally in the script section, I have used jQuery to write the logic for all the functionalities. I have made use of all the above-mentioned functions to accomplish this. This functionality is achieved using jQuery event handlers, which are triggered when the corresponding button is clicked.
Example
The following is the complete code which we are going to be using in this example −
<!DOCTYPE html> <html> <head> <title>How to Add Edit and Delete Table Row in jQuery?</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <style> table{ border: 2px solid black } td{ padding: 2px } </style> </head> <body> <h4>How to Add Edit and Delete Table Row in jQuery? </h4> <table id="my-table"> <tr> <td>Original Row</td> <td>Data</td> </tr> <tr> <td>Original Row</td> <td>Data</td> </tr> </table> <br> <button id="add-btn">Add Row</button> <button id="remove-btn">Remove Row</button> <button id="edit-btn">Edit Row</button> <script> $(document).ready(function(){ $("#add-btn").click(function(){ var newRow = "<tr><td>New Row</td><td>Data</td></tr>"; $("#my-table").append(newRow); }); $("#remove-btn").click(function(){ $("#my-table tr:last").remove(); }); $("#edit-btn").click(function(){ let rows=$("#my-table").find("tr") let idx=Math.floor(Math.random()*rows.length) rows.eq(idx).find("td").eq(0).text("Edited Row") }); }); </script> </body> </html>
Conclusion
In this article we got introduced to number of methods in jQuery, namely they were append(), find() and remove(). We saw how we can use a combination of these methods provided by jQuery alongside some DOM manipulation techniques to add, edit and delete table rows dynamically.