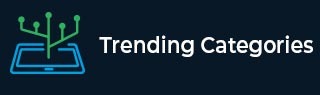
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to add a Video Player in ReactJS?
Tp add a Video Player in ReactJS, we first need to install a video player library such as 'react-player' or 'react-video-js'. Next, we import the library and use the designated component in our JSX code. Lastly, we provide the necessary props such as the video source and any customization options to the component.
Let us first understand what ReactJS is. React is a JavaScript library for building user interfaces. It makes it easy to create interactive UIs. Design simple views for each state in your application, and React will efficiently update and render the right components when your data changes. You can also build encapsulated components that manage their own state, then compose them to make complex UIs.
Both small and large, complicated applications can be created with ReactJS. It offers a basic yet reliable feature set to get a web application off the ground. It is easy to master both contemporary and legacy applications and is a faster method of coding a functionality. React provides high quantity of ready-made components readily available.
Now, let’s discuss the approach for doing the same.
To get started first create a new ReactJS app and run it on our dev server like this −
npx create-react-app video-app cd video-app npm start
Approach
Install the 'react-player' package −
npm install react-player
Import the 'react-player' component in your component file −
import ReactPlayer from 'react-player'
Add the 'react-player' component to the JSX code where you want the video player to appear −
<ReactPlayer url={'path/to/video'} controls={true} />
Make sure the video file is properly imported or referenced in the project.
Run the application and the video player should appear on the page.
You can customize the player with different props such as width, height, controls, loop and more.
You can also use the "onPlay" and "onPause" events to track the state of the video player −"
<ReactPlayer url={'path/to/video'} controls={true} onPlay={() => console.log('video is playing')} onPause={() => console.log('video is paused')} />
You can also use the "ref" prop to access the player's state and methods in the component −
const playerRef = useRef(null); <ReactPlayer ref={playerRef} url={'path/to/video'} controls={true} />
Example
PlayerComponent.js
import ReactPlayer from 'react-player'; import React, { useRef } from 'react'; const VIDEO_PATH = 'https://youtu.be/0BIaDVnYp2A'; function PlayerComponent() { const playerRef = useRef(null); return ( <div> <ReactPlayer ref={playerRef} url={VIDEO_PATH} controls={true} /> </div> ) }; export default PlayerComponent;
index.js
import React, { StrictMode } from 'react'; import { createRoot } from 'react-dom/client'; import PlayerComponent from './PlayerComponent'; const rootElement = document.getElementById('root'); const root = createRoot(rootElement); root.render( <StrictMode> <PlayerComponent /> </StrictMode> );
Output
