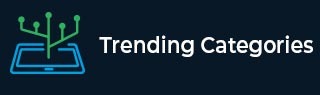
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to add a search box inside a responsive navigation menu?
To add a search box, use the input type text on a web page. Set the search box inside the navigation menu. Let us first set how to create a responsive navigation menu and place the search box.
Set the Navigation Menu and Search box in it
To begin with, use the <nav> to create a navigation menu. The links are set using the <a> element. Set input type="text" for the search box. This adds the search box inside the navigation menu −
<nav> <a class="links selected" href="#"> <i class="fa fa-fw fa-home"></i> Home </a> <a class="links" href="#"> <i class="fa fa-fw fa-user"></i> Login </a> <a class="links" href="#"> <i class="fa fa-user-circle-o" aria-hidden="true"></i> Register </a> <a class="links" href="#"> <i class="fa fa-fw fa-envelope"></i> Contact Us </a> <a class="links" href="#"> <i class="fa fa-info-circle" aria-hidden="true"></i> More Info </a> <input type="text" placeholder="Search Here.." /> </nav>
Place the Navigation Links
The menu links are styled and placed in the center. The underline is removed using the text-decoration property with the value none −
.links { display: inline-block; text-align: center; padding: 14px; color: rgb(178, 137, 253); text-decoration: none; font-size: 17px; }
Style the Search box
The search box is placed on the right using the float property with the value right.
input[type="text"] { float: right; padding: 6px; margin-top: 8px; margin-right: 8px; font-size: 17px; }
Make the Navigation Menu Responsive
The Media Queries forms the responsiveness of the navigation menu. Media Queries are used when you need to set a style to different devices such as tablet, mobile, desktop, etc. When the screen size gets smaller than 830px, the display is set to block for the links −
@media screen and (max-width: 830px) { .links { display: block; }
Example
The following is the code to add a search box inside a responsive navigation menu −
<!DOCTYPE html> <html lang="en" > <head> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Document</title> <link href="https://stackpath.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css" rel="stylesheet" integrity="sha384-wvfXpqpZZVQGK6TAh5PVlGOfQNHSoD2xbE+QkPxCAFlNEevoEH3Sl0sibVcOQVnN" crossorigin="anonymous" /> <style> body { margin: 0px; margin-top: 10px; padding: 0px; } nav { width: 100%; background-color: rgb(39, 39, 39); overflow: auto; height: auto; } .links { display: inline-block; text-align: center; padding: 14px; color: rgb(178, 137, 253); text-decoration: none; font-size: 17px; } .links:hover { background-color: rgb(100, 100, 100); } input[type="text"] { float: right; padding: 6px; margin-top: 8px; margin-right: 8px; font-size: 17px; } .selected { background-color: rgb(0, 18, 43); } @media screen and (max-width: 830px) { .links { display: block; } input[type="text"] { display: block; width: 100%; margin: 0px; border-bottom: 2px solid rgb(178, 137, 253); text-align: center; } } </style> </head> <body> <nav> <a class="links selected" href="#"> <i class="fa fa-fw fa-home"></i> Home </a> <a class="links" href="#"> <i class="fa fa-fw fa-user"></i> Login </a> <a class="links" href="#"> <i class="fa fa-user-circle-o" aria-hidden="true"></i> Register </a> <a class="links" href="#"> <i class="fa fa-fw fa-envelope"></i> Contact Us </a> <a class="links" href="#"> <i class="fa fa-info-circle" aria-hidden="true"></i> More Info </a> <input type="text" placeholder="Search Here.." /> </nav> </body> </html>