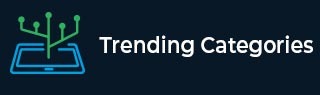
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to add a new element to HTML DOM in JavaScript?
In this article we are going to discuss how to add a new element to HTML DOM in JavaScript.
The Document object provides a method createElement() to create an element and appendChild() method to add it to the HTML DOM. Following are the steps involved in creating HTML DOM −
Step 1 − To insert an element into HTML DOM, firstly, we need to create an element and append to HTML DOM. The document.createElement() is used to create the HTML element. The syntax to create an element is shown below.
document.createElement(tagName[, options]);
Where,
tagName is the name of the tag to be created. The tag is of <p> type.
options param is an optional element object.
Step 2 − After creation of a tag, we need to create a text to assign to the tag. The syntax to create a text node is shown below.
Document.createTextNode("String");
Step 3 − After creating the text, we need to add the text to the element <p> type and thus finally adding to the div tag. The syntax to append the element to a tag is shown below.
appendChild(parameter);
Example 1
In the following example, initially the div section consists of only 2 texts. But later on, one more text is created and added to the div section as shown in the output.
<html> <body> <div id="new"> <p id="p1">Tutorix</p> <p id="p2">Tutorialspoint</p> </div> <script> var tag = document.createElement("p"); var text = document.createTextNode("Tutorix is the best e-learning platform"); tag.appendChild(text); var element = document.getElementById("new"); element.appendChild(tag); </script> </body> </html>
On executing the above code, the following output is generated.
Example 2
The following is an example program on how to add an element to HTML DOM.
<!DOCTYPE html> <html> <body> <h2>JavaScript HTML DOM</h2> <p>How to add a new element to HTML DOM</p> <div id="div1"> <p id="p1">Introduction.</p> <p id="p2">Conclusion.</p> </div> <script> const para = document.createElement("p"); const node = document.createTextNode("The end."); para.appendChild(node); const element = document.getElementById("div1"); const child = document.getElementById("p2"); element.appendChild(para,child); </script> </body> </html>
On executing the above code, the following output is generated.
Example 3
The following is an example program on how to add an element to HTML DOM. Here, the insertBefore() method is used instead of append method to add the element to the div tag.
<!DOCTYPE html> <html> <body> <h2>JavaScript HTML DOM</h2> <p>How to add a new element to HTML DOM</p> <div id="div1"> <p id="p1">Introduction.</p> <p id="p2">Conclusion.</p> </div> <script> const para = document.createElement("p"); const node = document.createTextNode("To begin with..."); para.appendChild(node); const element = document.getElementById("div1"); const child = document.getElementById("p2"); element.insertBefore(para,child); </script> </body> </html>
On executing the above code, the following output is generated.