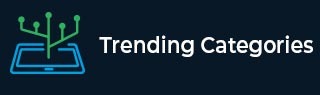
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How to access the JSON fields, arrays and nested objects of JsonNode in Java?
A JsonNode is Jackson's tree model for JSON and it can read JSON into a JsonNode instance and write a JsonNode out to JSON. To read JSON into a JsonNode with Jackson by creating ObjectMapper instance and call the readValue() method. We can access a field, array or nested object using the get() method of JsonNode class. We can return a valid string representation using the asText() method and convert the value of the node to a Java int using the asInt() method of JsonNode class.
In the below example, we can access JSON fields, arrays and nested objects of JsonNode.
Example
import com.fasterxml.jackson.databind.*; import java.io.*; public class ParseJSONNodeTest { public static void main(String args[]) { String jsonStr = "{ \"name\" : \"Raja\", \"age\" : 30," + " \"technologies\" : [\"Java\", \"Scala\", \"Python\"]," + " \"nestedObject\" : { \"field\" : \"value\" } }"; ObjectMapper objectMapper = new ObjectMapper(); try { JsonNode node = objectMapper.readValue(jsonStr, JsonNode.class); JsonNode nameNode = node.get("name"); String name = nameNode.asText(); System.out.println(name); JsonNode ageNode = node.get("age"); int age = ageNode.asInt(); System.out.println(age); JsonNode array = node.get("technologies"); JsonNode jsonNode = array.get(1); String techStr = jsonNode.asText(); System.out.println(techStr); JsonNode child = node.get("nestedObject"); JsonNode childField = child.get("field"); String field = childField.asText(); System.out.println(field); } catch (IOException e) { e.printStackTrace(); } } }
Output
Raja 30 Scala value
Advertisements