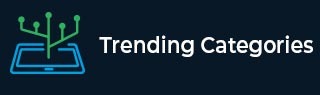
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How semaphore is used to implement mutual exclusion?
A semaphore is a shared variable which is used to implement mutual exclusion between system processes. It is mainly helpful to solve critical section problems and is a technique to achieve process synchronization.
There are two types of semaphores which are as follows −
Binary semaphore − Can take only two values, 0 or 1 which means at a time only one process can enter into the critical section. Semaphore is initialized to 1.
Counting semaphore − Can take any non-negative value N which means at a time at most N processes can enter into CS. Semaphore is initialized to N.
The critical section is surrounded by P and V operations as follows −
P(s)
CS
V(s)
Each of these operations is defined below −
Wait(P) − Whenever a process enters into CS, first it executes P operation where it decreases semaphore value and if after that s>=0 then enters into CS otherwise added to the waiting queue.
P(Semaphore s) { s = s - 1; if (s < 0) { block(p); } }
Signal(V) − When a process exists CS operation V is performed which increases the value of semaphore indicating another process can enter into CS which is currently blocked by P operation.
V(Semaphore s) { s = s + 1; if (s >= 0) { wakeup(p); } }
Let us see how the lock variable is used to introduce the mutual exclusion −
It uses a similar mechanism as semaphore but at a time only one process can enter into a critical section and uses lock variable to implement synchronization as below −
while(lock != 0); Lock = 1; //critical section Lock = 0;
It checks if the lock is equal to 0 then sets the lock to 1 indicating that lock is occupied and then enters into CS. If the lock is not 0, then wait until it is available. When exiting CS set lock back to 0 indicating lock is available and another process can enter into CS.
The difference between locks and semaphores is that locks can be implemented in user mode whereas semaphores are implemented in kernel mode. Also, locks allow only one process to enter into CS but semaphore can allow multiple processes to enter into CS. In short, semaphore is the generalization of locks.