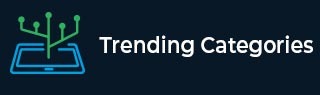
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How does Selenium Webdriver handle SSL certificate in Chrome?
We can handle SSL certificate with Selenium webdriver in Chrome browser. A SSL is the standardized protocol used to create a connection between the browser and server.
The information exchanged via a SSL certificate is encrypted and it verifies if the information is sent to the correct server. It authenticates a website and provides protection from hacking.
An untrusted SSL certificate error is thrown if there are problems in the SSL certificate. We shall receive such an error while we launch a website. In Chrome, we use the ChromeOptions class to work with SSL certificates.
We shall create an instance of this class and set the capability - setAcceptInsecureCerts to true. Finally, this property of the Chrome browser shall be passed to the webdriver object.
Syntax
ChromeOptions c = new ChromeOptions(); c.setAcceptInsecureCerts(true);
Example
import org.openqa.selenium.By; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; import org.openqa.selenium.WebDriver; public class SSLErrorChrome { public static void main(String[] args) throws IOException { //object of ChromeOptions ChromeOptions c = new ChromeOptions(); //set browser properties c.setAcceptInsecureCerts(true); System.setProperty("webdriver.chrome.driver", "C:\Users\ghs6kor\Desktop\Java\chromedriver.exe"); // pass browser option to webdriver WebDriver driver = new ChromeDriver(c); //implicit wait driver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS); //URL launch driver.get("application url to be entered"); driver.quit(); } }