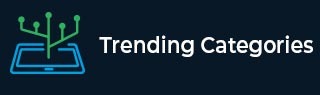
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How does Recursion work in Ruby Programming?
A function that calls itself directly or indirectly is called a recursive function, and the corresponding function is referred to as a recursive function. Recursion makes the process easier and it definitely reduces the compilation time.
We will try to understand the concept of recursion in Ruby with the help of a very simple example.
Let's suppose we are given an array and we want to print the product of all the elements of the array, in order to do that, we have two choices, we can do it iteratively or we can do it recursively.
Example 1
Let's first do it iteratively. Consider the code shown below where the multiplication of each element is returned by the function.
# Iterative program to print multiplication of array elements def iterativeMultiplication(arr) mul = 1 arr.each do |num| mul *= num end puts mul end iterativeMultiplication([1, 2, 3, 4, 5])
Output
It will generate the following output −
120
Example 2
Now let's do it recursively. Consider the recursive code shown below.
# Recursive program to print multiplication of array elements def recursiveMultiplication(arr) return 1 if arr.length == 0 arr[0] * recursiveMultiplication(arr[1..-1]) end puts recursiveMultiplication([1,2,3,4,5])
Output
It will generate the following output −
120
Example 3
Now let's explore one more example, which is a very famous recursive problem. The problem is to print the famous nth Fibonacci number.
Consider the code shown below −
# print fibonacci nth number in ruby def printFib( n ) return n if ( 0..1 ).include? n ( printFib( n - 1 ) + printFib( n - 2 ) ) end puts printFib( 5 )
Output
It will generate the following output −
5