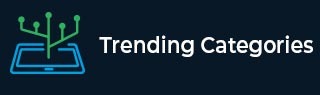
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How do you upcast and downcast the same object in Java?
Converting one data type to others in Java is known as casting.
Up casting − If you convert a higher datatype to lower datatype, it is known as narrowing (assigning higher data type value to the lower data type variable).
Example
import java.util.Scanner; public class NarrowingExample { public static void main(String args[]){ char ch = (char) 67; System.out.println("Character value of the given integer: "+ch); } }
Output
Character value of the given integer: C
Down casting − If you convert a lower datatype to a higher data type, it is known as widening (assigning lower data type value to the higher data type variable).
Example
public class WideningExample { public static void main(String args[]){ char ch = 'C'; int i = ch; System.out.println(i); } }
Output
Integer value of the given character: 67
upcasting and downcasting the same object
Similarly, you can also cast/convert an object of one class type to others. But these two classes should be in an inheritance relation. Then,
If you convert a Super class to subclass type it is known as narrowing in terms of references (Subclass reference variable holding an object of the superclass).
Sub sub = (Sub)new Super();
If you convert a Subclass to Superclass type it is known as widening in terms of references (superclass reference variable holding an object of the subclass).
Super sup = new Sub();
Example
The following Java program demonstrates how to upcast and down cast the same object.
class Person{ public String name; public int age; Person(){} public Person(String name, int age){ this.name = name; this.age = age; } public void displayPerson() { System.out.println("Data of the Person class: "); System.out.println("Name: "+this.name); System.out.println("Age: "+this.age); } } public class Student extends Person { public String branch; public int Student_id; Student(){} public Student(String name, int age, String branch, int Student_id){ super(name, age); this.branch = branch; this.Student_id = Student_id; } public void displayStudent() { System.out.println("Data of the Student class: "); System.out.println("Name: "+super.name); System.out.println("Age: "+super.age); System.out.println("Branch: "+this.branch); System.out.println("Student ID: "+this.Student_id); } public static void main(String[] args) { Person person = new Person(); Student student = new Student("Krishna", 20, "IT", 1256); //Up casting person = student; person.displayPerson(); //only super class methods //Down casting student = (Student) person; student.displayPerson(); student.displayStudent(); } }
Output
Data of the Person class: Name: Krishna Age: 20 Data of the Person class: Name: Krishna Age: 20 Data of the Student class: Name: Krishna Age: 20 Branch: IT Student ID: 1256