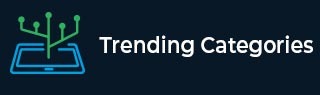
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How do you implement persistent objects in Python?
To implement persistent objects in Python, use the following libraries.
- shelve
- pickle
The shelve module
A “shelf” is a persistent, dictionary-like object. The difference with “dbm” databases is that the values (not the keys!) in a shelf can be essentially arbitrary Python objects — anything that the pickle module can handle. This includes most class instances, recursive data types, and objects containing lots of shared sub-objects.
It is having some key methods −
shelve.open() − Open a persistent dictionary. The filename specified is the base filename for the underlying database. As a side-effect, an extension may be added to the filename and more than one file may be created. By default, the underlying database file is opened for reading and writing.
shelve.sync() − Write back all entries in the cache if the shelf was opened with writeback set to True. Also empty the cache and synchronize the persistent dictionary on disk, if feasible. This is called automatically when the shelf is closed with close().
shelve.close() − Synchronize and close the persistent dict object.
The pickle module
The pickle module implements binary protocols for serializing and de-serializing a Python object structure.
Pickling is the process through which a Python object hierarchy is converted into a byte stream. To serialize an object hierarchy, you simply call the dumps() function.
Unpickling is the inverse operation. A byte stream from a binary file or bytes-like object is converted back into an object hierarchy. To de-serialize a data stream, you call the loads() function.
Pickle Module Functions
The following are the functions provided by the pickle module.
pickle.dump() − Write the pickled representation of the object to the open file object file.
pickle.dumps() − Return the pickled representation of the object as a bytes object, instead of writing it to a file.
pickle.load() − Read the pickled representation of an object from the open file object file.
pickle.loads() − Return the reconstituted object hierarchy of the pickled representation data of an object
Example
First, the pickle module is imported −
import pickle
We have created the following input to be pickled.
my_data = { 'BMW', 'Audi', 'Toyota', 'Benz'}
The demo.pickle file is created. This same .pickle file is pickled with the above list.
with open("demo.pickle","wb") as file_handle: pickle.dump(my_data, file_handle, pickle.HIGHEST_PROTOCOL)
Now, unpickle the above pickled file and get the input values back.
with open("demo.pickle","rb") as file_handle: res = pickle.load(file_handle) print(res_data)
Let us now see the complete example.
import pickle # Input Data my_data = { 'BMW', 'Audi', 'Toyota', 'Benz'} # Pickle the input with open("demo.pickle","wb") as file_handle: pickle.dump(my_data, file_handle, pickle.HIGHEST_PROTOCOL) # Unpickle the above pickled file with open("demo.pickle","rb") as file_handle: res = pickle.load(file_handle) print(my_data) # display the output
Output
set(['Benz', 'Toyota', 'BMW', 'Audi'])