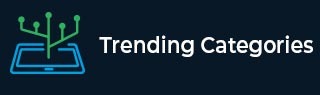
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How do threads communicate with each other in Java?
Inter-thread communication involves the communication of threads with each other. The three methods that are used to implement inter-thread communication in Java.
wait()
This method causes the current thread to release the lock. This is done until a specific amount of time has passed or another thread calls the notify() or notifyAll() method for this object.
notify()
This method wakes a single thread out of multiple threads on the current object’s monitor. The choice of thread is arbitrary.
notifyAll()
This method wakes up all the threads that are on the current object’s monitor.
Example
class BankClient { int balAmount = 5000; synchronized void withdrawMoney(int amount) { System.out.println("Withdrawing money"); balAmount -= amount; System.out.println("The balance amount is: " + balAmount); } synchronized void depositMoney(int amount) { System.out.println("Depositing money"); balAmount += amount; System.out.println("The balance amount is: " + balAmount); notify(); } } public class ThreadCommunicationTest { public static void main(String args[]) { final BankClient client = new BankClient(); new Thread() { public void run() { client.withdrawMoney(3000); } }.start(); new Thread() { public void run() { client.depositMoney(2000); } }.start(); } }
Output
Withdrawing money The balance amount is: 2000 Depositing money The balance amount is: 4000
Advertisements