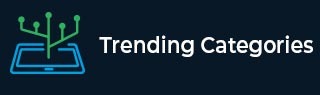
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How do I iterate over a sequence in reverse order in Python?
Python Sequences includes Strings, Lists, Tuples, etc. We can merge elements of a Python sequence using different ways. Let’s see some examples of iteration over a List in reverse order.
Iterate in Reverse Order using while loop
Example
In this example, we have a List as a sequence and iterate it in reverse order using the while loop −
# Creating a List mylist = ["Jacob", "Harry", "Mark", "Anthony"] # Displaying the List print("List = ",mylist) # Length - 1 i = len(mylist) - 1 # Iterate in reverse order print("Display the List in Reverse order = ") while i >= 0 : print(mylist[i]) i -= 1
Output
List = ['Jacob', 'Harry', 'Mark', 'Anthony'] Display the List in Reverse order = Anthony Mark Harry Jacob
Iterate in Reverse Order using for loop
Example
In this example, we have a List as a sequence and iterate it in reverse order using the for loop −
# Creating a List mylist = ["Jacob", "Harry", "Mark", "Anthony"] # Displaying the List print("List = ",mylist) # Iterate in reverse order print("Display the List in Reverse order = ") for i in range(len(mylist) - 1, -1, -1): print(mylist[i])
Output
List = ['Jacob', 'Harry', 'Mark', 'Anthony'] Display the List in Reverse order = Anthony Mark Harry Jacob
Iterate in Reverse Order using reversed()
Example
In this example, we have a List as a sequence and iterate it in reverse order using the reversed() method −
# Creating a List mylist = ["Jacob", "Harry", "Mark", "Anthony"] # Displaying the List print("List = ",mylist) # Iterate in reverse order using reversed() print("Display the List in Reverse order = ") [print (i) for i in reversed(mylist)]
Output
List = ['Jacob', 'Harry', 'Mark', 'Anthony'] Display the List in Reverse order = Anthony Mark Harry Jacob
Iterate in Reverse Order using Negative Indexing
Example
In this example, we have a List as a sequence and iterate it in reverse order using negative indexing −
# Creating a List mylist = ["Jacob", "Harry", "Mark", "Anthony"] # Displaying the List print("List = ",mylist) # Iterate in reverse order using negative indexing print("Display the List in Reverse order = ") [print (i) for i in mylist[::-1]]
Output
List = ['Jacob', 'Harry', 'Mark', 'Anthony'] Display the List in Reverse order = Anthony Mark Harry Jacob
Advertisements