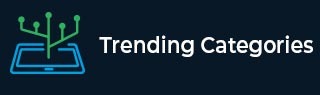
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How do I get length of list of lists in Java?
List provides a method size() to get the count of elements currently present in the list. To get size of each list, we can use iterate through each item as list and add their sizes to get the count of all elements present in the list of lists. In this example, we are using streams to achieve the same.
Syntax
int size()
Returns the number of elements in this list. If this list contains more than Integer.MAX_VALUE elements, returns Integer.MAX_VALUE.
Example
The following example shows how to check length of list of lists using size() method and streams.
package com.tutorialspoint; import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class CollectionsDemo { public static void main(String[] args) { List<Integer> list1 = new ArrayList<>(Arrays.asList(1,2,3)); List<Integer> list2 = new ArrayList<>(Arrays.asList(4,5,6,7)); List<Integer> list3 = new ArrayList<>(Arrays.asList(8,9)); List<List<Integer>> list = new ArrayList<>(Arrays.asList(list1, list2, list3)); int count = list.stream().mapToInt(i -> i.size()).sum(); System.out.println("Total elements: " + count); } }
Output
This will produce the following result −
Total elements: 9
Advertisements