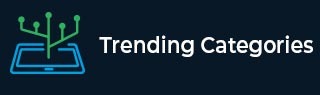
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How do I generate random floats in C++?
In C or C++, we cannot create random float directly. We can create random floats using some trick. We will create two random integer values, then divide them to get random float value.
Sometimes it may generate an integer quotient, so to reduce the probability of that, we are multiplying the result with some floating point constant like 0.5.
Example
#include <iostream> #include <cstdlib> #include <ctime> using namespace std; main() { srand((unsigned int)time(NULL)); float a = 5.0; for (int i=0;i<20;i++) cout << (float(rand())/float((RAND_MAX)) * a) << endl; }
Output
2.07648 4.3115 1.31092 2.22465 2.17292 1.48381 1.91137 0.56505 2.24326 4.44517 3.1695 2.39067 1.89062 4.35881 4.17524 0.189673 1.87521 1.76916 2.3217 2.20481
Advertisements