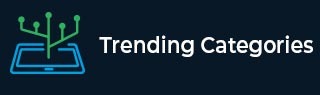
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How do I convert an integer to binary in JavaScript?
Are you looking for ways to convert an integer to binary in JavaScript? If so, this article is for you! In this article, we will discuss the various methods available for converting an integer into its binary equivalent using JavaScript.
We’ll look at how a number can be broken down into individual bits and manipulated accordingly. Converting an integer to binary is simply changing the integer value into a binary value. For example, assume we have passed two integer values, 22 and 45, respectively, as:
var x = 22; var y = 45;
Now, if we perform the conversion of these two integer values to a binary value, the console statement for var x will print 10110, and the console statement for var y will print 101101.
console.log(dec2bin(22)); // 10110 console.log(dec2bin(45));//101101
Example
In the following example, we are running a script using, ‘if-while’ condition to convert the integer value into binary value.
<!DOCTYPE html> <html> <body> <script> function toBinary(n) { n = Number(n); if (n == 0) return '0'; var r = ''; while (n != 0) { r = ((n&1)?'1':'0') + r; n = n >>> 1; } return r; } document.write(toBinary(5)+"<br>"); document.write(toBinary(22)+"<br>"); document.write(toBinary(45)+"<br>"); </script> </body> </html>
When the script gets executed, it will generate an output consisting of binary values, which are converted from integer values by an event that gets triggered on executing the above script.
Example
Consider the following example, where we are using the script to convert integer value to binary value.
<!DOCTYPE html> <html> <body> <script> function convertto(dec) { return (dec >>> 0).toString(2); } document.write(convertto(111)+"<br>"); document.write(convertto(222)+"<br>"); document.write(convertto(333)+"<br>"); </script> </body> </html>
On running the above script, the output window will pop up, displaying the binary values on the webpage obtained by changing the integer values caused by an event that gets triggered on running the script.
Example
Let’s consider the following example, where we are using the ‘if-else’ condition to convert integer to binary.
<!DOCTYPE html> <html> <body> <script> function changeto(number, res = "") { if (number < 1) if (res === "") return "0" else return res else return changeto(Math.floor(number / 2), number % 2 + res) } document.write(changeto(99)+"<br>") document.write(changeto(88)+"<br>") document.write(changeto(77)+"<br>") </script> </body> </html>
When the script gets executed, the event gets triggered, which converts the given integer values into binary values and displays them on the webpage.
Example
Execute the below to observe how the integer value getting changed to binary value.
<!DOCTYPE html> <html> <body> <script> const int2dec = v => { if (v === 0) return ''; let remainder = v % 2; let quotient = (v - remainder) / 2; if (remainder === 0) { return int2dec(quotient) + '0'; } else { return int2dec(quotient) + '1'; } } const betterint2dec = v => { if (v === 0) return '0'; if (v < 0) return '-' + int2dec(-v); return int2dec(v); } document.write(betterint2dec(20)); </script> </body> </html>
On running the above script, the event gets triggered, and displays the binary value on the webpage occurred on changing the integer value.