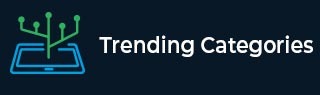
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How do I add a class to a given element using Javascript?
In JavaScript, there are various approaches to add a class to an element. We can use the .className attribute or the .add() method to add a class name to the specific element.
The class attribute can be used in CSS to perform some tasks for the elements with the parallel class name.
Using the className Property
We can use this property to add a class to an HTML element without restoring its existing class. This property can be used to execute the value of the class attribute of an element.
If a class is already declared for an element, and we have to add a new class name to the same element then we should declare a new class by inserting a space before writing the name of the class. (i.e document.getElementById("").className = " newClass");
Syntax
You can set the value to this property as shown below −
element.className += "newClass";
Where newClass specifies the element’s class name. for applying more than one class, it needs to separate by space.
The following statement returns the value of the className property.
element.className;
This property holds a string value representing the names of the class or list of classes separated with a space.
Example 1
In the following example, we are adding the class name using the .className property.
<!DOCTYPE html> <html lang="en"> <head> <title>class</title> </head> <style> .CSS{ color: blueviolet; font-style: italic; background-color: rgb(227, 171, 171); } </style> <body> <button onclick="addClass()">AddClass</button> <h3 id="heading" >Tutorialspoint easy to learn</h3> <script> function addClass(){ var add_class = document.getElementById("heading"); add_class.className += "CSS"; } </script> </body> </html>
After clicking on button(AddClass). The heading looks like the below image.
Example 2
In the following example, we can also do the same by using the below code, and it will give the same output.
<!DOCTYPE html> <html lang="en"> <head> <title>class</title> </head> <style> .CSS{ color: blueviolet; font-style: italic; background-color: rgb(227, 171, 171); } </style> <body> <button onclick="addClass()">AddClass</button> <h3 id="heading" >Tutorialspoint easy to learn</h3> <script> function addClass(){ var add_class = document.getElementById("heading").className = "CSS"; } </script> </body> </html>
Using the add() Method
This method is useful for adding a class name to the preferred or selected element. Following is the syntax of this method −
Element.classList.add("newClass");
Example 1
In the following example, we are using the add() method to add a class name to the paragraph element having id = p1
<!DOCTYPE html> <html> <head> <title>add class name using JavaScript</title> <style> .newClass { font-size: 30px; background-color: rgb(149, 237, 136); color: rgb(19, 21, 19); border: 2px solid rgb(58, 35, 35); } </style> </head> <body> <h1>Hello Tutorialspoint :)</h1> <p id="p1">Welcome to the tutorialspoint.com</p> <p>Click the following button to see the effect.</p> <button onclick="addClass()">Add Class</button> <script> function addClass() { var add_class = document.getElementById("p1"); add_class.classList.add("newClass"); } </script> </body> </html>
Example 2
In the following example, we are adding a new class to the existing class.
<!DOCTYPE html> <html lang="en"> <head> <title>class</title> </head> <style> .CSS { color: blueviolet; font-style: italic; background-color: rgb(227, 171, 171); } </style> <body> <button onclick="addClass()">AddClass</button> <h3 id="heading" class="head">Tutorialspoint easy to learn</h3> <script> function addClass() { var add_class = (document.getElementById("heading").className = " CSS"); //add_class.className += "CSS"; } </script> </body> </html>
Before clicking the button (Add class). Here class is not changing.
After clicking the button (Add Class). Here class is changing from head to CSS.