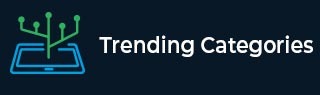
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How do I access the serial (RS232) port in Python?
To access the serial port in Python, use the pyserial module, which Python Serial Port Extension for Win32, OSX, Linux, BSD, Jython, IronPython.
Let us see the features -
- Access to the port settings through Python properties.
- Support for different byte sizes, stop bits, parity and flow control with RTS/CTS and/or Xon/Xoff.
- Working with or without receive timeout.
- The files in this package are 100% pure Python.
- The port is set up for binary transmission. No NULL byte stripping, CR-LF translation etc.
To install pyserial, use pip
pip install pyserial
At first import the required libraries.
import time import serial
Then, configure the serial connections.
ser = serial.Serial( port='Enter Port', baudrate=9600, parity=serial.PARITY_ODD, stopbits=serial.STOPBITS_TWO, bytesize=serial.SEVENBITS )
Get keyboard input using the input()
input = 1 while 1: # get keyboard input input = input(">> ") if input == 'exit': ser.close() exit()
If input is not equal to exit, send the character to the device using the write()
else: ser.write(input + '\r\n') out = ''
Wait one second before reading output.
time.sleep(1) while ser.inWaiting() > 0: out += ser.read(1) if out != '': print ">>" + out
Advertisements