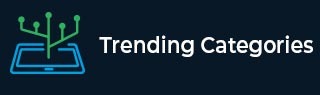
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How can we update an existing JSON data using javax.json API in Java?
The JsonBuilderFactory interface is a factory to create JsonObjectBuilder instance and JsonObjectBuilder is a builder for creating JsonObject models from scratch. This interface initializes an empty JSON object model and provides methods to add name/value pairs to the object model and to return the resulting object. We can create a JsonObjectBuilder instance that can be used to build JsonObject using the createObjectBuilder() method.
Syntax
JsonObjectBuilder createObjectBuilder()
In the below example, We can update an existing JSON data with newly added data.
Example
import java.io.*; import javax.json.*; public class UpdateExistingJsonTest { public static void main(String[] args) throws Exception { String jsonString = "{\"id\":\"115\", \"name\":\"Raja\", \"address\":[{\"area\":\"Madhapur\", \"city\":\"Hyderabad\"}]}"; StringReader reader = new StringReader(jsonString); JsonReader jsonReader = Json.createReader(reader); System.out.println("Existing JSON: \n" + jsonString); StringWriter writer = new StringWriter(); JsonWriter jsonWriter = Json.createWriter(writer); JsonObject jsonObject = jsonReader.readObject(); JsonBuilderFactory jsonBuilderFactory = Json.createBuilderFactory(null); JsonObjectBuilder jsonObjectBuilder = jsonBuilderFactory.createObjectBuilder(); for(String key : jsonObject.keySet()) { jsonObjectBuilder.add(key, jsonObject.get(key)); } jsonObjectBuilder.add("Contact Number", "9959984000"); jsonObjectBuilder.add("Country", "India"); jsonObject = jsonObjectBuilder.build(); jsonWriter.writeObject(jsonObject); System.out.println("new JSON: \n" + jsonObject); } }
Output
Existing JSON: {"id":"115", "name":"Raja", "address":[{"area":"Madhapur", "city":"Hyderabad"}]} new JSON: {"id":"115","name":"Raja","address":[{"area":"Madhapur","city":"Hyderabad"}],"Contact Number":"9959984000","Country":"India"}
Advertisements