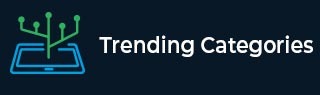
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How can we create an exception filter to handle unhandled exceptions in C#
ASP.NET WebAPI?
An exception filter is executed when a controller method throws any unhandled exception that is not an HttpResponseException exception. The HttpResponseException type is a special case, because it is designed specifically for returning an HTTP response.
Exception filters implement the System.Web.Http.Filters.IExceptionFilter interface. The simplest way to write an exception filter is to derive from the System.Web.Http.Filters.ExceptionFilterAttribute class and override the OnException method.
Below is a filter that converts NotFiniteNumberException exceptions into HTTP status code 416, Requested Range Not Satisfiable.
ExceptionFilterAttribute −
Example
using System; using System.Net; using System.Net.Http; using System.Web.Http.Filters; namespace DemoWebApplication.Controllers{ public class ExceptionAttribute : ExceptionFilterAttribute{ public override void OnException(HttpActionExecutedContext context){ if (context.Exception is NotFiniteNumberException){ context.Response = new HttpResponseMessage(HttpStatusCode.RequestedRangeNotSatisfiable); } } } }
Controller ActionMethod −
Example
using DemoWebApplication.Models; using System; using System.Collections.Generic; using System.Linq; using System.Web.Http; namespace DemoWebApplication.Controllers{ [Exception] public class StudentController : ApiController{ List<Student> students = new List<Student>{ new Student{ Id = 1, Name = "Mark" }, new Student{ Id = 2, Name = "John" } }; public Student Get(int id){ if(id <= 0){ throw new NotFiniteNumberException("The Id is not valid"); } var studentForId = students.FirstOrDefault(x => x.Id == id); return studentForId; } } }
So let us test the above ExceptionAttribute by passing id = 0 for the controller action method.
The ExceptionAttribute can be registered by any of te following approaches.
Decorate Action with exception filter.
[Exception] public IHttpActionResult Get(int id){ Return Ok(); }
Decorate Controller with exception filter.
[Exception] public class StudentController : ApiController{ public IHttpActionResult Get(int id){ Return Ok(); } }
Register exception globally in WebApiConfig.cs.
public static class WebApiConfig{ public static void Register(HttpConfiguration config){ config.Filters.Add(new ExceptionAttribute()); } }