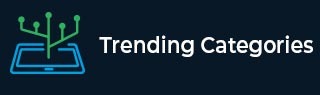
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How can we add an underscore before each capital letter inside a java String?
Using the StringBuffer class
To add underscore before each capital letter in a String using StringBuffer −
Create an empty StringBuffer object.
The isUpperCase() method of Character class accepts a character and verifies whether it is in upper case, if so, this method returns true. Using this method, verify each character in the String.
In case of upper case letter append underscore before it, using the append() method.
Example
public class Adding_BeforeCapital { public static void main(String args[]) { String str = "HelloHowAreYouWelcome"; StringBuffer sb = new StringBuffer(); for (int i = 0; i < str.length(); i++) { if(Character.isUpperCase(str.charAt(i))) { sb.append("_"); sb.append(str.charAt(i)); } else { sb.append(str.charAt(i)); } } String result = sb.toString(); System.out.println(result); } }
Output
_Hello_How_Are_You_Welcome
Using Regular Expression
The replaceAll() method of the String class accepts two strings representing a regular expression and a replacement String and replaces the matched values with given String.
Example
public class Adding_BeforeCapital { public static void main(String args[]) { String str = "HelloHowAreYouWelcome"; String result = str.replaceAll("()([A-Z])", "$1_$2"); System.out.println(result); } }
Output
_Hello_How_Are_You_Welcome
Advertisements