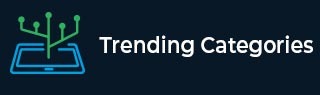
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How can I use a variable inside a Dockerfile CMD?
Introduction
Using variables in a Dockerfile CMD can greatly improve the flexibility and maintainability of container configuration. Hardcoding values in a CMD instruction can make it difficult to update or modify the container's configuration in the future. Using variables allows for more dynamic and reusable configurations.
Prerequisites
The prerequisites for using variables inside a Dockerfile CMD depend on the method you choose to use −
Using shell expansion − No specific prerequisites are required.
Using the ENV instruction − No specific prerequisites are required.
Using the ARG instruction − No specific prerequisites are required.
Passing variable at runtime − No specific prerequisites are required, but you should have a running Docker daemon and have the Docker command-line tool installed.
Using the SHELL instruction − You should have a shell installed on your system that is compatible with the syntax used in the SHELL instruction.
Using the --build-arg flag during the build of the image − You should have Docker installed on your system.
Using the runtime variable in the compose file and passing it to the container during the docker-compose up command − You should have Docker and Docker-compose installed on your system
Using the ENV_FILE instruction in the compose file to pass environment variables from a file to the container − You should have Docker and Docker-compose installed on your system and a file with key-value pairs containing environment variables
It's also important to note that in all cases, the variable should be defined before it's used in the CMD instruction, whether that's through the ENV or ARG instruction, shell expansion, or runtime flag.
Ways to use a variable inside a Dockerfile CMD
Using shell expansion − You can include variables in the CMD instruction by using shell expansion. The syntax is $VARIABLE or ${VARIABLE}. For example −
ENV VARIABLE=default CMD echo “Printing the value of the VARIABLE : $VARIABLE”
Using the ENV instruction − You can use the ENV instruction in a Dockerfile to set an environment variable and reference it in the CMD instruction using the $VARIABLE syntax. For example −
ENV VARIABLE=default CMD echo “Printing the value of the VARIABLE : $VARIABLE”
You can also pass environment variables at runtime using the -e flag in the docker run command −
docker run -e VARIABLE=new_value myimage
Using the ARG instruction − You can use the ARG instruction in a Dockerfile to set a variable during build time, and reference it in the CMD instruction using the $VARIABLE syntax. For example −
ARG VARIABLE=default CMD echo “Printing the value of the VARIABLE : $VARIABLE”
You can pass the variable value during the build using --build-arg flag −
docker build --build-arg VARIABLE=new_value -t myimage .
Passing variable at runtime − You can pass a variable at runtime using the -e flag in the docker run command and reference it in the CMD instruction using the $VARIABLE syntax
Using the SHELL instruction − You can use the SHELL instruction to specify a different shell for command expansion, and reference variables in the CMD instruction using the shell's variable syntax. For example −
SHELL ["bash", "-c"] ENV VARIABLE=default CMD echo “Printing the value of the VARIABLE : $VARIABLE”
Using the --build-arg flag during the build of the image
docker build --build-arg VARIABLE=new_value -t myimage .
Using the runtime variable in the compose file and passing it to the container during the docker-compose up command
version: "3.8" services: web: build: . environment: - VARIABLE=${VARIABLE}
Using the ENV_FILE instruction in the compose file to pass environment variables from a file to the container.
version: "3.8" services: web: build: . env_file: - .env
And in the .env file −
VARIABLE=new_value
In all the above examples, you can reference the variable in the CMD instruction using the $VARIABLE syntax.
CMD echo “Printing the value of the VARIABLE : $VARIABLE”
It's important to note that in the case of ENV_FILE, the file should contain key-value pairs, one pair per line, where the key is the name of the environment variable, and the value is the value that should be assigned to the variable.
Understanding the syntax for using variables in a Dockerfile CMD
Docker provides several ways to use variables in a CMD instruction, such as using ENV, ARG, or passing them at runtime.
ENV instruction sets environment variables and can be referenced in the CMD instruction using the syntax $VARIABLE. For example, the following Dockerfile sets an environment variable VARIABLE with the value default and references it in the CMD instruction −
FROM alpine ENV VARIABLE=default CMD echo “Printing the value of the VARIABLE : $VARIABLE”
and when running the container, you can pass the value of the variable at runtime using -e flag −
docker run -e VARIABLE=new_value myimage
ARG instruction is used to define variables during the build process and can be referenced in the CMD instruction using the syntax ${VARIABLE}. For example, the following Dockerfile sets an ARG VARIABLE with the default value default and references it in the CMD instruction −
FROM alpine ARG VARIABLE=default CMD echo "The value of VARIABLE is: ${VARIABLE}"
and when building the image, you can pass the value of the variable using --build-arg flag −
docker build --build-arg VARIABLE=new_value -t myimage .
It is also possible to pass variables at runtime using the docker run command. for example −
docker run --env VARIABLE=new_value myimage
Best practices for using variables in a Dockerfile CMD
When using variables in a Dockerfile CMD, it's important to keep in mind the following best practices −
Use environment variables for sensitive information like passwords and tokens, and make sure to not include them in the image.
Be mindful of the performance and try to minimize the number of environment variables used in the CMD instruction.
Use variables in a way that allows for easy updates and modifications to the container configuration in the future.
Conclusion
In conclusion, using variables in a Dockerfile CMD can greatly improve the flexibility and maintainability of container configuration. By using the ENV, ARG, or passing at runtime options, you can create more dynamic and configurable containers. Additionally, by following best practices such as using environment variables for sensitive information and being mindful of performance, you can ensure a secure and reliable container deployment.