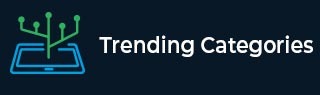
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How can I pass parameters in computed properties in VueJS?
The difference between a computed property and a method is that computed properties are cached, these properties only change when their dependency change. A method will be evaluated every time it is being called. The only useful situation is when the user wants the getter and needs to have the same parameterized. The same situation occurs in vuex also.
Example
Copy and paste the below code snipped in your Vue project and run the Vue project. You shall see the below output on the browser window.
FileName - app.js
Directory Structure -- $project/public -- app.js
// Retrieving the computed properties from parameters new Vue({ el: '#container', data() { return { name: 'John', lastname: 'Wick' } }, filters: { prepend: (name, lastname, prefix) => { return `${prefix} ${name} ${lastname}` } } });
FileName - index.html
Directory Structure -- $project/public -- index.html
<!DOCTYPE html> <html> <head> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> </head> <body> <div style="text-align: center;"> <h2> Passing Components in Computed Properties </h2> </div> <div id="container"> <h3 style="text-align: center;">{{ name, lastname | prepend('Gracias ! ') }}!</h3> </div> <script src='app.js'></script> </body> </html>
Run the following command to get the below output −
C://my-project/ > npm run serve
Complete Code with HTML
Now let’s create a HTML file with the help of the above app.js and index.html files. The below Program can be run as an HTML file.
<!DOCTYPE html> <html> <head> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> </head> <body> <div style="text-align: center;"> <h2> Passing Components in Computed Properties </h2> </div> <div id="container"> <h3 style="text-align: center;">{{ name, lastname | prepend('Gracias ! ') }}!</h3> </div> <script> // Retrieving the computed properties from parameters new Vue({ el: '#container', data() { return { name: 'John', lastname: 'Wick' } }, filters: { prepend: (name, lastname, prefix) => { return `${prefix} ${name} ${lastname}` } } }); </script> </body> </html>
Example
The below example demonstrates how to pass parameters to a computed property in Vue.js −
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Passing parameters to Computed Properties</title> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> </head> <body> <div id="app"> <h2>Passing parameters to Computed Properties </h2> <div> <label for="first-name">First Name:</label> <input type="text" id="first-name" v-model="firstName"> </div> <div> <label for="last-name">Last Name:</label> <input type="text" id="last-name" v-model="lastName"> </div> <div> <label for="full-name">Full Name:</label> <span id="full-name">{{ getFullName('Hello') }}</span> </div> </div> <script> new Vue({ el: '#app', data: { firstName: '', lastName: '', }, computed: { getFullName: function () { return function (prefix) { return prefix + ' ' + this.firstName + ' ' + this.lastName; } }, }, }); </script> </body> </html>
Here we define two data properties - firstName and lastName. These properties are bound to the input fields using the v-model directive.
We've also defined a computed property called fullName that concatenates the firstName and lastName data properties with a space between them.
Finally, we're rendering the computed property fullName in a span element with the id attribute set to full-name. When the user types in the input fields, the computed property is automatically updated and the full name is displayed in the span element.