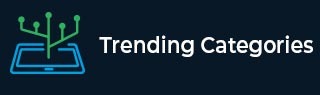
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How can I identify when a Button is released in Tkinter?
In Tkinter, events are generally called by buttons or keys. Whenever the user presses an assigned key or clicks an assigned button, the events get executed. To execute the events, we can bind a button or a key with the callback function.
Consider an application where we need to trigger an event whenever we release the mouse button. This can be achieved by passing the <Button Release> parameter in the bind(<ButtonRelease>, callback) method.
Example
# Import the required libraries from tkinter import * # Create an instance of tkinter frame or window win=Tk() # Set the size of the window win.geometry("700x350") # Define a function on mouse button clicked def on_click(event): label["text"]="Hello, There!" def on_release(event): label["text"]="Button Released!" # Crate a Label widget label=Label(win, text="Click anywhere..", font=('Calibri 18 bold')) label.pack(pady=60) win.bind("<ButtonPress-1>", on_click) win.bind("<ButtonRelease-1>", on_release) win.mainloop()
Output
If we run the above code, it will display a window with a label widget.
Now, click anywhere in the window to see the message on the screen that will get updated when we release the mouse button.
When you release the mouse button, it will display the following message −
Advertisements