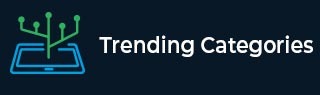
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How can I handle multiple keyboard keys using Selenium Webdriver?
We can handle multiple keyboard keys in Selenium webdriver by using the method Keys.chord. The multiple keyboard keys to be handled are passed as parameters to this method.
The return type of the Keys.chord method is a string and can be applied to an element with the help of the sendKeys method. For example, in order to perform a select operation on text entered inside an edit box we require the keys ctrl+a to be pressed simultaneously.
Syntax
String k = Keys.chord(Keys.CONTROL, "A"); driver.findElement(By.name("q")).sendKeys(k);
Example
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; import org.openqa.selenium.Keys; public class MultipleKeys{ public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "C:\Users\ghs6kor\Desktop\Java\chromedriver.exe"); WebDriver driver = new ChromeDriver(); //implicit wait driver.manage().timeouts().implicitlyWait(5, TimeUnit.SECONDS); //URL launch driver.get("https://www.google.com/"); //identify element WebElement l = driver.findElement(By.name("q")); l.sendKeys("Java"); // method Keys.chord String k = Keys.chord(Keys.CONTROL, "A"); //handling multiple keys l.sendKeys(k); } }
Output
Advertisements