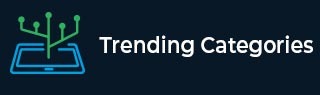
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How can I get H1 innerText in JavaScript without the innerText of its child?
Getting the inner text of an HTML element is a common task when developing web applications. In JavaScript, it can be done using the innerText property of the HTMLElement object. However, this only returns the text within that particular element and not any of its child elements.
If you need to get just the H1 tag's innertext without including its child elements' innertext, then there are several ways to do so. This article will discuss some methods for getting an H1 tag's innertext in JavaScript without including its child elements' innertext.
The rendered text content of a node, and its descendants is represented by the innerText property of the HTMLElement interface. As a getter, it simulates the text; the user would obtain if they used the cursor to highlight the element's contents and then copied them to the clipboard.
Syntax
Following is the syntax for innertext in JavaScript.
element.innerText
let’s look into the following example to understand more about how can I get h1 innertext in JavaScript without the innertext of its child.
Example
In the following example we are running the script to get innertext in JavaScript.
<!DOCTYPE html> <html> <body> <div class="Bike"> <div class="Car"> <label for="name"><b>Actual Text:</b></label> <h1> This is BMW<span> That Was Ducati </span></h1> </div> </div> <h3>Innertext:</h3> <script> const h1 = document.querySelector('div.Car h1'); const text = h1.childNodes[0].textContent; document.write(text); </script> </body> </html>
Example
Considering the following example where we are running script to get h1 innertext along adding some extra message.
<!DOCTYPE html> <html> <body> <div class="tutorial"> <div class="tutorial1"> <label for="actual"><b>Actual Text:</b></label><br> <h1>Welcome <span>to the Tutorialpoint</span> HELLO </h1> </div> </div> <h1>Innertext:</h1> <script> const h1 = document.querySelector('div.tutorial h1'); const el = h1.childNodes; let result = ""; for(i=0;i<el.length;i++){ if(el[i].nodeName == '#text'){ result+=el[i].textContent; } } document.write(result); </script> </body> </html>
Example
Let’s look into the another example to obtain innertext in JavaScript
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css"> <script src="https://code.jquery.com/jquery-1.12.4.js"></script> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script> <body> <div class="first-header"> <div class="second-header"> <h1> This is h1 tag........<span>This is span tag inside h1 tag.......... </span></h1> </div> </div> </body> <script> var valueOfh1 = document.querySelector('div.second-header h1'); var value = valueOfh1.childNodes[0].textContent; document.write(value); </script> </html>