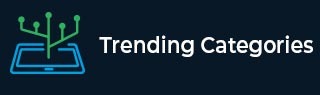
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
How can I consistently remove the default text from an input element with Selenium?
We can consistently remove the default text from an input element with Selenium. The clear method is used to remove the values currently present in an edit box or a text area.
The Keys.chord method along with sendKeys can also be used. The Keys.chord method allows you to pass more than one key at once. The group of keys or strings are passed as arguments to the method.
First of all, pass Keys.CONTROL and a as arguments to the Keys.chord method. The whole string is then passed as an argument to the sendKeys method. Last, we have to pass Keys.DELETE to the sendKeys method.
Let's take the below edit field where we will remove the default text.
Example
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class RemDefaultVal{ public static void main(String[] args) { System.setProperty("webdriver.chrome.driver","C:\Users\ghs6kor\Desktop\Java\chromedriver.exe"); WebDriver driver = new ChromeDriver(); driver.get("https://www.tutorialspoint.com/index.htm"); // identify element WebElement m = driver.findElement(By.id("gsc−i−id1")); // delete default value with clear() m.clear(); driver.quit(); } }
Example
import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.Keys; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; import java.util.concurrent.TimeUnit; public class RemDefaultValKeys{ public static void main(String[] args) { System.setProperty("webdriver.chrome.driver","C:\Users\ghs6kor\Desktop\Java\chromedriver.exe"); WebDriver driver = new ChromeDriver(); driver.get("https://www.tutorialspoint.com/index.htm"); // identify element WebElement m = driver.findElement(By.id("gsc−i−id1")); // sending Ctrl+A by the Keys.Chord String st = Keys.chord(Keys.CONTROL, "a"); l.sendKeys(st); // DELETE key sent l.sendKeys(Keys.DELETE); driver.quit(); } }
Advertisements