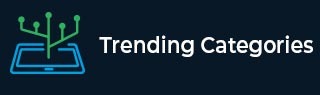
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Highest and lowest value difference of array JavaScript
In this problem we have to create an algorithm to get the highest and lowest value difference of an array with the help of Javascript functionality. So we will solve the problem with for loop and initialization of highest and lowest values to Infinity.
Understanding the logic of the problem statement
The problem statement is asking to write the code for getting the highest and lowest value difference of the array.
So for solving this task we will initialize two variables, these variables will store the highest and lowest values. And then iterate through every element of the array with the help of a loop. Then we will check that the element is higher than the current value of highest and update as required. Then check for the lowest value and update as required. After getting both the values we will calculate the difference of them, which is the required result.
For example: if we have an array as [1 ,2 ,3 , 4, 5, 6], as we can see in this array the highest element is 6 and lowest element is 1 and the difference between these two values is 6 - 1 = 5. So the resultant output will be 5.
Algorithm
Here's the step by step process of the algorithm:
Step 1: Initialize two variables for storing the highest and lowest values of the array. And set values as -Infinity and Infinity. These values represent the guaranteed lower and higher values than any other number in the array.
Step 2: Iterate through each item of the array with the help of a for loop. For each element in the array we will check if the element is higher than the current value of highest. If this condition is true then update the new value of highest.
Step 3: And check if the element is lower than the current value of the lowest then update to the new value of the lower value.
Step 4: Calculate the difference between the highest and lowest values by subtracting lowest from highest. And store this value in another variable and give it a name as difference.
Step 5: At the end show the output as the highest, lowest and the difference to the console with the usage of console.log statements.
Example
//define array const array = [1, 5, 10, 2, 4, 6, 9]; //define highest and lowest values let highest = -Infinity; let lowest = Infinity; //iterate the array elements for (let i = 0; i < array.length; i++) { if (array[i] > highest) { highest = array[i]; } if (array[i] < lowest) { lowest = array[i]; } } //difference between highest and lowest const difference = highest - lowest; console.log("Highest value:", highest); console.log("Lowest value:", lowest); console.log("Difference:", difference);
Output
Highest value: 10 Lowest value: 1 Difference: 9
Complexity
The complexity for getting the highest and lowest element difference of an array using the given process is O(n) where n is the length of the given array elements. Because the algorithm is iterating through the elements of the array one time and comparing the current highest and lowest values. So the number of iterations is proportional to the number of elements in the array so the resulting complexity is linear.
Conclusion
We can solve the given problem by iterating the array elements with a for loop. And finding the highest and lowest values from the array and calculating the difference between these two values. And showed the required result in the console.