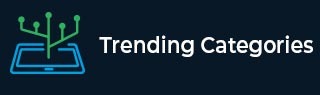
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Higher-Order Function in Golang
Higher-order functions are a powerful feature of modern programming languages, including Golang. In this article, we will discuss what higher-order functions are and how they can be used in Golang.
What is a Higher-Order Function?
A higher-order function is a function that takes one or more functions as arguments and/or returns a function as its result. This means that higher-order functions can be used to create new functions that are tailored to specific needs, making code more efficient and reusable.
Higher-Order Function Example in Golang
Let's consider a simple example to understand how higher-order functions work in Golang. Suppose we have a program that needs to calculate the square of a number. We can define a function to do this as follows −
func square(x int) int { return x * x }
Now suppose we need to calculate the cube of a number. We can define a new function to do this −
func cube(x int) int { return x * x * x }
This works, but it's not very efficient. We can use a higher-order function to create a more efficient solution −
Example
package main import ( "fmt" ) func power(fn func(int) int) func(int) int { return func(x int) int { return fn(x) } } func square(x int) int { return x * x } func cube(x int) int { return x * x * x } func main() { squareFunc := power(square) cubeFunc := power(cube) fmt.Println(squareFunc(2)) // Output: 4 fmt.Println(cubeFunc(2)) // Output: 8 }
Output
4 8
In this example, we have defined a higher-order function called power. This function takes a function as its argument and returns a new function that applies the given function to its argument. We can use this higher-order function to create new functions for calculating the square and cube of a number. By using the power function, we can avoid repeating the code for calculating the square and cube of a number, making our code more efficient and easier to maintain.
Benefits of Higher-Order Functions in Golang
The use of higher-order functions in Golang has several benefits −
Reusability − Higher-order functions allow us to create new functions that are tailored to specific needs, making code more reusable.
Flexibility − Higher-order functions can be used to create more flexible and adaptable code that can be easily customized to different use cases.
Efficiency − By avoiding the repetition of code, higher-order functions can make code more efficient, reducing the number of lines of code and improving performance.
Conclusion
Higher-order functions are a powerful feature of modern programming languages, including Golang. By using higher-order functions, we can create new functions that are tailored to specific needs, making code more efficient and reusable. In this article, we have discussed what higher-order functions are and how they can be used in Golang. By following the examples provided, you can learn how to use higher-order functions to write efficient and scalable programs in Golang.